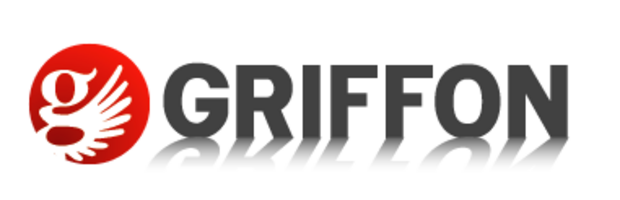
1. Introduction
Developing desktop/RIA applications on the JVM is a hard task. You have to make choices upfront during application design that might complicate the implementation, compromising the user experience; not to mention the amount of configuration needed.
RCP solutions like Eclipse RCP and NetBeans RCP are great for developing desktop applications, not so much for RIAs and applets. Griffon is a framework inspired by Grails, whose aim is to overcome the problems you may encounter while developing all these types of applications. It brings along popular concepts like
-
Convention over Configuration
-
Don’t Repeat Yourself (DRY)
-
Pervasive MVC
-
Testing supported "out of the box"
This documentation will take you through getting started with Griffon and building desktop/RIA applications with the Griffon framework.
Credits and Acknowledgements
This guide is heavily influenced by the Grails Guide. It simply would not have been possible without the great efforts made by: Graeme Rocher, Peter Ledbrook, Marc Palmer, Jeff Brown and their sponsor: SpringSource. The Griffon team would like to thank them all (and the Grails community too!) for making such a great framework and bringing the fun back to programming applications.
2. Getting Started
This chapter will help you get started with Griffon by explaining how to download and install the project, how to use Griffon from within your favorite IDE, and how to write your first application.
2.1. Environment Setup
The following section outlines the minimum environment requirements to get started with Griffon.
2.2. Console Example
2.2.1. Creating a Project
The first step is to get Lazybones installed on your system. The easiest way to achieve this goal is to install GVM first
$ curl -s get.gvmtool.net | bash
Install the latest version of Lazybones with the following command
$ gvm install lazybones
Next, add the official Griffon Lazybones templates repository to your Lazybones
configuration. Edit $USER_HOME/.lazybones/config.groovy
and paste the following
content
bintrayRepositories = [
"griffon/griffon-lazybones-templates",
"pledbrook/lazybones-templates"
]
We’re now ready to create the project. You can list all available templates with the following command
$ lazybones list
Available templates in griffon/griffon-lazybones-templates:
griffon-javafx-java
griffon-javafx-groovy
griffon-lanterna-java
griffon-lanterna-groovy
griffon-pivot-java
griffon-pivot-groovy
griffon-swing-java
griffon-swing-groovy
griffon-plugin
Notice that template names follow a naming convention identifying the main UI toolkit and main programming language. Alright, let’s create a simple project using Swing as main UI toolkit and Groovy as main language
$ lazybones create griffon-swing-groovy console
Creating project from template griffon-swing-groovy (latest) in 'console'
Define value for 'group' [org.example]: console
Define value for 'version' [0.1.0-SNAPSHOT]:
Define value for 'package' [console]:
Define value for 'griffonVersion' [2.0.0.BETA3]:
2.2.2. Project Layout
Take a moment to familiarize yourself with the standard Griffon project layout. Every Griffon project shares the same layout, making it easy to dive in as artifacts are located in specific directories according to their responsibilities and behavior.
console
├── griffon-app
│ ├── conf
│ ├── controllers
│ ├── i18n
│ ├── lifecycle
│ ├── models
│ ├── resources
│ ├── services
│ └── views
└── src
├── integration-test
│ └── groovy
├── main
│ ├── groovy
│ └── resources
└── test
├── groovy
└── resources
Griffon uses "convention over configuration" approach to enable a fast development pace. This typically means that the name and location of files is used instead of explicit configuration, hence you need to familiarize yourself with the directory structure provided by Griffon.
Here is a breakdown and links to relevant sections:
-
griffon-app
- top level directory for Groovy sources.-
conf
- Configuration sources. -
models
- Models. -
views
- Views. -
controllers
- Controllers. -
services
- Services. -
resources
- Images, properties files, etc.
-
-
src
- Supporting sources.-
main
- Other Groovy/Java sources.
-
-
test
- Unit tests. -
integration-test
- Integration tests.
Here’s an screenshot of the finished application running to give you an idea of what we’re aiming at with this example . A small Groovy script has been executed, you can see the result on the bottom right side
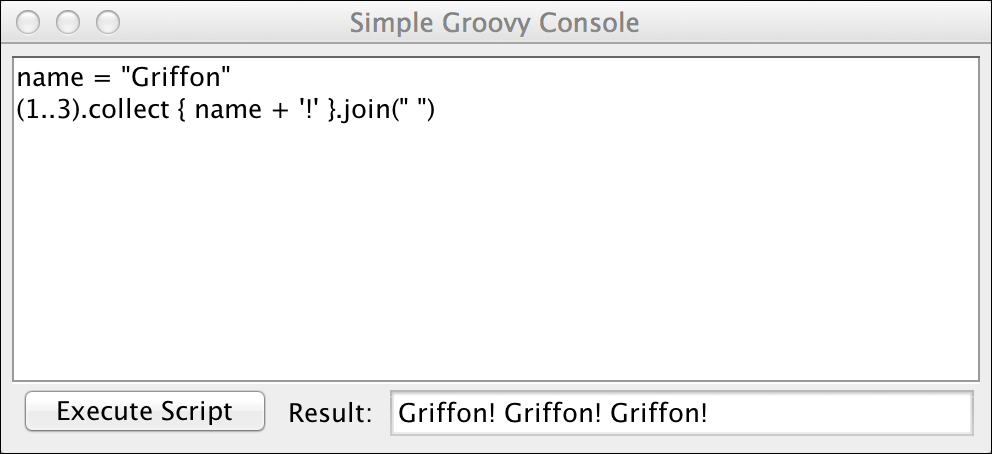
Gradle
The following listing shows the Gradle build file generated by the Lazybones template
buildscript {
repositories {
jcenter()
}
dependencies {
classpath 'org.codehaus.griffon:gradle-griffon-plugin:1.0.0.BETA2'
classpath 'net.saliman:gradle-cobertura-plugin:2.2.4'
classpath 'org.kt3k.gradle.plugin:coveralls-gradle-plugin:0.4.1'
classpath 'nl.javadude.gradle.plugins:license-gradle-plugin:0.9.0'
}
}
apply plugin: 'groovy'
apply plugin: 'org.codehaus.griffon.griffon'
griffon {
disableDependencyResolution = false
includeGroovyDependencies = true
version = '2.0.0.BETA3'
toolkit = 'swing
}
apply from: 'gradle/publishing.gradle'
apply from: 'gradle/code-coverage.gradle'
apply from: 'gradle/code-quality.gradle'
apply from: 'gradle/integration-test.gradle'
mainClassName = 'console.Launcher'
dependencies {
compile "org.codehaus.griffon:griffon-guice:${griffon.version}"
runtime('log4j:log4j:1.2.17') {
exclude group: 'ant', module: 'ant-nodeps'
exclude group: 'ant', module: 'ant-junit'
exclude group: 'ant-contrib', module: 'ant-contrib'
}
runtime 'org.slf4j:slf4j-log4j12:1.7.7'
testCompile "org.codehaus.griffon:griffon-fest-test:${griffon.version}"
}
Alright, let’s get started with the code. We’ll visit the Model first.
Model
The model for this application is simple: it contains properties that hold the script to be
evaluated and the results of the evaluation. Make sure you paste the following code into
griffon-app/models/console/ConsoleModel.groovy
.
package console
import griffon.core.artifact.GriffonModel
import griffon.metadata.ArtifactProviderFor
import griffon.transform.Observable
@ArtifactProviderFor(GriffonModel)
class ConsoleModel {
String scriptSource (1)
@Observable Object scriptResult (2)
@Observable boolean enabled = true (3)
}
1 | Holds the script’s text |
2 | Holds the result of the script’s execution |
3 | Enable/disable flag |
Griffon Models are not domain classes like the ones you find in Grails; they’re more akin to presentation models, and as such, they’re used to transfer data between Views and Controllers.
Controller
The controller is also trivial: throw the contents of the script from the model at an Evaluator
,
then store the result back into the model. Make sure you paste the following code into
griffon-app/controllers/console/ConsoleController.groovy
.
package console
import griffon.core.artifact.GriffonController
import griffon.metadata.ArtifactProviderFor
import javax.inject.Inject
@ArtifactProviderFor(GriffonController)
class ConsoleController {
def model (1)
@Inject
Evaluator evaluator (2)
void executeScript() { (3)
model.enabled = false
def result
try {
result = evaluator.evaluate(model.scriptSource) (4)
} finally {
model.enabled = true
model.scriptResult = result (5)
}
}
}
1 | MVC member injected by MVCGroupManager |
2 | Injected by JSR 330 |
3 | Controller action; automatically executed off the UI thread |
4 | Evaluate the script |
5 | Write back result to Model |
The Griffon framework will inject references to the other portions of the MVC triad if fields
named model
, view
, and controller
are present in the Model, Controller or View. This allows
us to access the view widgets and the model data if needed. Any other class members annotated with
@Inject
participate in dependency injection as laid out by JSR 330, in this case the controller
will get an instance of Evaluator
if a suitable implementation is bound.
The executeScript
3 method will be used later in the View in combination
with a button. You may notice that there’s no explicit threading management. All Swing developers
know they must obey the Swing Rule: long running computations must run outside of the EDT; all UI
components should be queried/modified inside the EDT. It turns out Griffon is aware of this rule,
making sure an action is called outside of the EDT by default; all bindings made to UI components
via the model will be updated inside the EDT 5. We’ll setup the bindings in
the next listing.
We must create a Module
in order to bind Evaluator
.
These are the required class definitions
package console
public interface Evaluator {
Object evaluate(String input)
}
package console
class GroovyShellEvaluator implements Evaluator {
private GroovyShell shell = new GroovyShell()
@Override
Object evaluate(String input) {
shell.evaluate(input)
}
}
package console
import griffon.core.injection.Module
import griffon.inject.DependsOn
import griffon.swing.SwingWindowDisplayHandler
import org.codehaus.griffon.runtime.core.injection.AbstractModule
import org.kordamp.jipsy.ServiceProviderFor
import static griffon.util.AnnotationUtils.named
@DependsOn('swing') (3)
@ServiceProviderFor(Module)
class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(Evaluator) (1)
.to(GroovyShellEvaluator)
.asSingleton()
bind(SwingWindowDisplayHandler) (2)
.withClassifier(named('defaultWindowDisplayHandler'))
.to(CenteringWindowDisplayHandler)
.asSingleton()
}
}
1 | Binding definition |
2 | Overriding an existing binding |
3 | Loaded after swing module |
Modules can define several bindings, even override existing bindings. In our particular
case we defined a binding 1 for Evaluator
and overrode a
binding 2 for SwingWindowDisplayHandler
. The latter is
supplied by the swing
module thus we must mark it as a dependency 3
in our module definition. The implementation of our custom SwingWindowDisplayHandler
is quite trivial, as shown by the following snippet
package console
import org.codehaus.griffon.runtime.swing.DefaultSwingWindowDisplayHandler
import javax.annotation.Nonnull
import java.awt.Window
import static griffon.swing.support.SwingUtils.centerOnScreen
class CenteringWindowDisplayHandler extends DefaultSwingWindowDisplayHandler {
@Override
void show(@Nonnull String name, @Nonnull Window window) {
centerOnScreen(window)
super.show(name, window)
}
}
This handler is only concerned with centering the window on the screen before showing it.
View
The view classes contain the visual components for your application. Please paste the following code
into griffon-app/views/console/ConsoleView.groovy
.
package console
import griffon.core.artifact.GriffonView
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonView)
class ConsoleView {
def builder (1)
def model (1)
void initUI() {
builder.with {
actions {
action(executeScriptAction, (2)
enabled: bind { model.enabled })
}
application(title: application.configuration['application.title'],
pack: true, locationByPlatform: true, id: 'mainWindow',
iconImage: imageIcon('/griffon-icon-48x48.png').image,
iconImages: [imageIcon('/griffon-icon-48x48.png').image,
imageIcon('/griffon-icon-32x32.png').image,
imageIcon('/griffon-icon-16x16.png').image]) {
panel(border: emptyBorder(6)) {
borderLayout()
scrollPane(constraints: CENTER) {
textArea(text: bind(target: model, 'scriptSource'), (3)
enabled: bind { model.enabled }, (2)
columns: 40, rows: 10)
}
hbox(constraints: SOUTH) {
button(executeScriptAction) (4)
hstrut(5)
label('Result:')
hstrut(5)
textField(editable: false,
text: bind { model.scriptResult }) (5)
}
}
}
}
}
}
1 | MVC member injected by MVCGroupManager |
2 | Bind enabled state from model |
3 | Bind script source to model |
4 | Apply controller action by convention |
5 | Bind script result from model |
The View contains a fairly straightforward SwingBuilder script. Griffon will execute these groovy
scripts in context of it’s CompositeBuilder
.
2.2.3. Running the application
Running the application requires you to execute the run
task if using Gradle
$ ./gradlew run
Or the exec:java
task if using Maven. Take special note that this task assumes classes
have been compiled already, so it’s best to pair it up with compile
for safe measure
$ mvn compile exec:java
Now that we know the basic structure of a Griffon application and how to run it we turn to testing.
2.2.4. Testing
It’s always a good idea to test out the code we write. It’s pretty easy to write tests for
regular components such as Evaluator
and GroovyShellEvaluator
, as they require little
to no external dependencies. Testing Griffon artifacts such as Controllers and Models on the
other hand requires a bit more of effort, but not much as shown by ConsoleControllerTest
package console
import griffon.core.artifact.ArtifactManager
import griffon.core.injection.Module
import griffon.core.test.GriffonUnitRule
import griffon.core.test.TestFor
import griffon.inject.DependsOn
import org.codehaus.griffon.runtime.core.injection.AbstractModule
import org.junit.Rule
import org.junit.Test
import javax.annotation.Nonnull
import javax.inject.Inject
import static com.jayway.awaitility.Awaitility.await
import static com.jayway.awaitility.Awaitility.fieldIn
import static java.util.concurrent.TimeUnit.SECONDS
import static org.hamcrest.Matchers.notNullValue
@TestFor(ConsoleController) (1)
class ConsoleControllerTest {
private ConsoleController controller (2)
@Inject
private ArtifactManager artifactManager (3)
@Rule
public final GriffonUnitRule griffon = new GriffonUnitRule() (4)
@Test
void testExecuteScriptAction() {
// given: (5)
ConsoleModel model = artifactManager.newInstance(ConsoleModel.class)
controller.model = model
// when: (6)
String input = 'var = "Griffon"'
model.scriptSource = input
controller.invokeAction('executeScript')
// then: (7)
await().atMost(2, SECONDS)
.until(fieldIn(model)
.ofType(Object)
.andWithName('scriptResult'),
notNullValue())
assert input == model.scriptResult
}
private static class EchoEvaluator implements Evaluator { (8)
@Override
Object evaluate(String input) {
input
}
}
@DependsOn('application')
private static class TestModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(Evaluator)
.to(EchoEvaluator)
.asSingleton()
}
}
@Nonnull
private List<Module> moduleOverrides() {
[new TestModule()]
}
}
1 | Indicate class under test |
2 | Injected by GriffonUnitRule given 1 |
3 | Injected by GriffonUnitRule via JSR 330 |
4 | Instantiates and configures a GriffonAplication for testing |
5 | Setup collaborators |
6 | Stimulus |
7 | Validate after waiting 2 seconds at most |
At the heart we have the @TestFor
1 annotation and a JUnit4 rule:
@GriffonUnitRule
4. These two key elements are responsible for
injecting the required behavior to the test case. @TestFor
identifies the type of component,
a Controller in this case, that’s under test; it assumes a suitable private field 2
to be defined in the testcase. This field is used to inject the instance under test.
The GriffonUnitRule
is responsible for bootstrapping a barebones application and putting
together all the required bindings. Notice that the test case can participate in dependency
injection too 3.
Running tests requires executing the test
task
$ ./gradlew test
A similar command can be invoked with Maven
$ mvn test
These are the basics for getting started with a Griffon project.
3. Application Overview
3.1. Configuration
It may seem odd that in a framework that embraces "convention-over-configuration" that we tackle this topic now, but since what configuration there is typically a one off, it is best to get it out the way.
3.1.1. Basic Configuration
For general configuration Griffon provides a file called griffon-app/conf/Config.groovy
.
This file uses Groovy’s ConfigSlurper which is very similar to Java properties files
except it is pure Groovy hence you can re-use variables and use proper Java types!
Here’s a typical configuration file
package sample.swing.groovy
application {
title = 'Swing + Groovy'
startupGroups = ['sample']
autoShutdown = true
}
mvcGroups {
// MVC Group for "sample"
'sample' {
model = 'sample.swing.groovy.SampleModel'
view = 'sample.swing.groovy.SampleView'
controller = 'sample.swing.groovy.SampleController'
}
}
You can define this file using Java too
package sample.swing.java;
import griffon.util.AbstractMapResourceBundle;
import griffon.util.CollectionUtils;
import javax.annotation.Nonnull;
import java.util.Map;
import static java.util.Arrays.asList;
public class Config extends AbstractMapResourceBundle {
@Override
protected void initialize(@Nonnull Map<String, Object> entries) {
CollectionUtils.map(entries)
.e("application", CollectionUtils.map()
.e("title", "Swing + Java")
.e("startupGroups", asList("sample"))
.e("autoShutdown", true)
)
.e("mvcGroups", CollectionUtils.map()
.e("sample", CollectionUtils.map()
.e("model", "sample.swing.java.SampleModel")
.e("view", "sample.swing.java.SampleView")
.e("controller", "sample.swing.java.SampleController")
)
);
}
}
Or if you prefer properties files then do the following
application.title = Swing + Groovy
application.startupGroups = sample
application.autoShutdown = true
mvcGroups.sample.model = sample.swing.groovy.SampleModel
mvcGroups.sample.view = sample.swing.groovy.SampleView
mvcGroups.sample.controller = sample.swing.groovy.SampleController
Take special note that this file must be placed under griffon-app/resources
instead.
The application’s runtime configuration is available through the configuration
property
of the application instance.
3.1.2. Internationalization Support
Configuration files are i18n aware which means you can append
locale specific strings to a configuration file, for example Config_de_CH.groovy
.
Locale suffixes are resolved from least to most specific; for a locale with
language = de, country = CH and variant = Basel the following files are loaded in order
-
Config.groovy
-
Config.properties
-
Config_de.groovy
-
Config_de.properties
-
Config_de_CH.groovy
-
Config_de_CH.properties
-
Config_de_CH_Basel.groovy
-
Config_de_CH_Basel.properties
The current java.util.Locale
is used to determine values for language, country and variant.
3.2. Metadata
The Griffon runtime keeps track of useful metadata that can be consumed by applications. The following sections describe them with more detail.
3.2.1. Application Metadata
Access to the application’s metadata file (application.properties
) is available by
querying the Metadata
singleton. Here’s a snippet of code that shows how to
setup a welcome message that displays the application’s name and version
package sample
import griffon.core.artifact.GriffonView
import griffon.core.env.Metadata
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonView)
class SampleView {
FactoryBuilderSupport builder
void initUI() {
Metadata meta = Metadata.current
builder.with {
application(pack: true,
title: application.configuration['application.title']) {
label "Hello, I'm ${meta['application.name']}-${meta['application.version']}"
}
}
}
}
There are also a few helpful methods found in Metadata
-
getApplicationName()
- same result asmeta[application.name]
-
getApplicationVersion()
- same result asmeta[application.version]
3.2.2. Application Environment
A Griffon application can run in several environments, default ones being
DEVELOPMENT
, TEST
and PRODUCTION
. Applications can inspect its current running
environment by means of the Environment
enum.
The following example enhances the previous one by displaying the current running environment
package sample
import griffon.core.artifact.GriffonView
import griffon.core.env.Metadata
import griffon.core.env.Environment
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonView)
class SampleView {
FactoryBuilderSupport builder
void initUI() {
Metadata meta = Metadata.current
builder.with {
application(pack: true,
title: application.configuration['application.title']) {
gridLayout cols: 1, rows: 2
label "Hello, I'm ${meta['application.name']}-${meta['application.version']}"
label "Current environment is ${Environment.current}"
}
}
}
}
The default environment is DEVELOPMENT. A different value can be specified by setting
a proper value for the griffon.env
System property. The Environment
class
recognizes the following aliases
-
dev
- short fordevelopment
. -
prod
- short forproduction
.
3.2.3. Griffon Environment
The GriffonEnvironment
gives you access to the following values
-
Griffon version
-
Griffon build date & time
-
JVM version
-
OS version
Here’s an example displaying all values
package sample
import griffon.core.artifact.GriffonView
import griffon.core.env.Metadata
import griffon.core.env.Environment
import griffon.metadata.ArtifactProviderFor
import static griffon.core.env.GriffonEnvironment.*
@ArtifactProviderFor(GriffonView)
class SampleView {
FactoryBuilderSupport builder
void initUI() {
Metadata meta = Metadata.current
builder.with {
application(pack: true,
title: application.configuration['application.title']) {
gridLayout cols: 1, rows: 6
label "Hello, I'm ${meta['application.name']}-${meta['application.version']}"
label "Current environment is ${Environment.current}"
label "Griffon version is ${getGriffonVersion()}"
label "Build date/time is ${getBuildDateTime()}"
label "JVM version is ${getJvmVersion()}"
label "OS version is ${getOsVersion()}"
}
}
}
}
3.3. Lifecycle
Every Griffon application goes through the same lifecycle phases no matter in which mode they are running, with the exception of applet mode where there is an additional phase due to the intrinsic nature of applets. The application’s lifecycle has been inspired by JSR-296, the Swing Application Framework.
Every phase has an associated lifecycle class that will be invoked at the appropriate
time. Class names match each phase name; you’ll find them inside griffon-app/lifecycle
.
3.3.1. Initialize
The initialization phase is the first to be called by the application’s life cycle. The application instance has just been created and its configuration has been read.
This phase is typically used to tweak the application for the current platform, including its Look & Feel.
The Initialize lifecycle handler will be called right after the configuration
has been read but before addons and managers are initialized.
|
3.3.2. Startup
This phase is responsible for instantiating all MVC groups that have been defined in the application’s configuration and that also have been marked as startup groups in the same configuration file.
The Startup lifecycle handler will be called after all MVC groups have been
initialized.
|
3.3.3. Ready
This phase will be called right after Startup
with the condition that no pending
events are available in the UI queue. The application’s main window will be displayed
at the end of this phase.
3.3.4. Shutdown
Called when the application is about to close. Any artifact can invoke the shutdown
sequence by calling shutdown()
on the GriffonApplication
instance.
The Shutdown lifecycle handler will be called after all ShutdownHandler s and
event handlers interested in the ShutdownStart event.
|
3.4. Modules
Module
s define components that can be injected using the JSR 330 API.
Module definitions are highly influenced by the Guice 3.x API however they do not
impose a strict dependency on Guice.
3.4.1. Module Definition
All modules must implement the Module
interface shown next
public interface Module {
@Nonnull
List<Binding<?>> getBindings();
void configure();
}
They also require to have a well defined name. This can be achieved by annotating the module
class with @javax.inject.Named
. If a value for @javax.inject.Named
is not defined then
one will be automatically calculated using the fully qualified class name. For example
package org.example;
import griffon.core.injection.Module;
import org.codehaus.griffon.runtime.core.injection.AbstractModule;
import org.kordamp.jipsy.ServiceProviderFor;
import javax.inject.Named;
@ServiceProviderFor(Module.class)
@Named
public class CustomModule extends AbstractModule {
@Override
protected void doConfigure() {
// bindings
}
}
Results in a module named org.example.custom
, where as the following definition
package org.example;
import griffon.core.injection.Module;
import org.codehaus.griffon.runtime.core.injection.AbstractModule;
import org.kordamp.jipsy.ServiceProviderFor;
import javax.inject.Named;
@ServiceProviderFor(Module.class)
@Named("custom")
public class CustomModule extends AbstractModule {
@Override
protected void doConfigure() {
// bindings
}
}
Results in a module simply called custom
. A module may depend on another module; when
that’s the case then the bindings of this module are processed after their dependencies. This
allows dependent modules to override bindings made by their dependencies. Module dependencies
are specified using the @griffon.inject.DependsOn
annotation, like so
import griffon.core.injection.Module;
import griffon.inject.DependsOn;
import org.codehaus.griffon.runtime.core.injection.AbstractModule;
import org.kordamp.jipsy.ServiceProviderFor;
import javax.inject.Named;
@ServiceProviderFor(Module.class)
@DependsOn("parent-module")
@Named
public class CustomModule extends AbstractModule {
@Override
protected void doConfigure() {
// bindings
}
}
3.4.2. Module Configuration
Modules perform their duty by defining bindings, of which there are 4 kinds
Bindings have an scope, either singleton
or prototype
. InstanceBinding
is the only
binding that has singleton
as implicit scope; this setting can’t be changed.
All bindings accept a classifier
or an classifier_type
. Classifiers are annotations that
have been annotated with @javax.inject.Qualifier
. You must specify one or the other but
not both; classifier
has precedence over classifier_type
.
Instance Binding
This binding is used to define explicit and eager singletons, for example
bind(Calculator.class)
.toInstance(new GroovyShellCalculator());
Target Binding
This binding enables you to specify the source type and target type. Typically the source is an interface while the target is a concrete implementation. If the target is omitted then the binding assumes the source to be a concrete type.
bind(Calculator.class)
.to(GroovyShellCalculator.class);
3.5. Shutdown Handlers
Applications have the option to let particular artifacts abort the shutdown sequence
and/or perform a task while the shutdown sequence is in process. Artifacts that desire
to be part of the shutdown sequence should implement the ShutdownHandler
interface and register themselves with the application instance.
The contract of a ShutdownHandler
is very simple
-
boolean canShutdown(GriffonApplication application)
- returnfalse
to abort the shutdown sequence. -
void onShutdown(GriffonApplication application)
- called if the shutdown sequence was not aborted.
ShutdownHandler
s will be called on the same order as they were registered.
3.6. Startup Arguments
Command line arguments can be passed to the application and be accessed by calling
getStartupArgs()
on the application instance. This will return a copy of the args
(if any) defined at the command line.
Here’s a typical example of this feature in development mode
package sample
import griffon.core.GriffonApplication
import griffon.core.event.EventHandler
class ApplicationEventHandler implements EventHandler {
void onBootstrapStart(GriffonApplication application) {
println application.startupArgs
}
}
Arguments must be defined in the build file if using Gradle
run {
args = ['one', 'two']
}
Running the application with run
command results in an output similar to the following
$ gradle run
:compileJava
:compileGroovy
:processResources
:classes
:run
// logging statements elided
[one, two]
4. The MVC Pattern
All Griffon applications operate with a basic unit called the MVC group. An MVC group is comprised of 3 member parts: Models, Views and Controllers. However it is possible to add (or even remove) members from an MVC group by carefully choosing a suitable configuration.
MVC groups configuration is setup in Config.groovy
located inside griffon-app/conf
(or
Config.java
if using Java as main language). This file holds an entry for every MVC group
that the application has (not counting those provided by Addons).
Here’s how a typical MVC group configuration looks like
mvcGroups {
// MVC Group for "sample"
'sample' {
model = 'sample.SampleModel'
view = 'sample.SampleView'
controller = 'sample.SampleController'
}
}
The definition order is very important, it determines the order in which each member
will be initialized. In the previous example both model
and view
will be initialized
before the controller
. Do not mistake initialization for instantiation, as initialization
relies on calling mvcGroupInit()
on the group member.
MVC group configurations accept a special key that defines additional configuration for that group, as it can be seen in the following snippet
mvcGroups {
// MVC Group for "sample"
'sample' {
model = 'sample.SampleModel'
view = 'sample.SampleView'
controller = 'sample.SampleController'
}
// MVC Group for "foo"
'foo' {
model = 'sample.FooModel'
view = 'sample.FooView'
controller = 'sample.FooController'
config {
key = 'bar'
}
}
}
Values placed under this key become available to MVC members during the call to
mvcGroupInit()
, as part of the arguments sent to that method. Here’s how
the FooController
can reach the key defined in the configuration
@ArtifactProviderFor(GriffonController)
class FooController {
void mvcGroupInit(Map<String, Object> args) {
println args.configuration.config.key
}
}
While being able to set additional values under this key is certainly an advantage
it would probably be better if those values could be mutated or tweaked, probably
treating them as variables, effectively making a group configuration work as a template.
For that we’ll have to discuss the MVCGroupManager
first.
4.1. The MVCGroupManager
This class is responsible for holding the configuration of all MVC groups no matter
how they were defined, which can be either in Config.groovy
or in an addon descriptor.
During the startup sequence an instance of MVCGroupManager
will be created
and initialized. Later the application will instruct this instance to create all startup
groups as required. MVCGroupManager
has a handful set of methods that deal with
MVC group configuration alone; however those that deal with group instantiation come
in 3 versions, with 2 flavors each (one Groovy, the other Java friendly).
Locating a group configuration is easily done by specifying the name you’re interested in finding
MVCGroupConfiguration configuration = application.mvcGroupManager.findConfiguration('foo')
Once you have a configuration reference you can instantiate a group with it by calling
any of the variants of the create
method
MVCGroupConfiguration configuration = application.mvcGroupManager.findConfiguration('foo')
MVCGroup group1 = configuration.create('foo1')
MVCGroup group2 = configuration.create('foo2', [someKey: 'someValue'])
// the following will make the group's id match its name
MVCGroup group3 = configuration.create()
MVCGroup group4 = configuration.create(someKey: 'someValue')
Be aware that creating groups with the same name is usually not a good idea. The
default MVCGroupManager
will complain when this happens and will automatically spit
out an exception. This behavior may be changed by setting a configuration key in Config.groovy
griffon.mvcid.collision = 'warning' // accepted values are 'warning', 'exception' (default)
The manager will log a warning and destroy the previously existing group before instantiating the new one when warning is the preferred strategy .
Now, even though you can create group instances based on their configurations the preferred
way is to call any of createMVCGroup()
,
buildMVCGroup()
or withMVCGroup()
methods. Any class annotated with the @griffon.transform.MVCAware
will also gain access to these methods.
Groups will be available by id regardless of how they were instantiated. You can ask
the MVCGroupManager
for a particular group at any time, for example
def g1 = application.mvcGroupManager.groups.foo1
def g2 = application.mvcGroupManager.findGroup('foo1')
def g3 = application.mvcGroupManager.foo1
assert g1 == g2
assert g1 == g3
It’s also possible to query all models, views, controllers and builders on their own. Say you’d want to inspect all currently instantiated models, this is how it can be done
application.mvcGroupManager.models.each { model ->
// do something with model
}
4.2. MVC Groups
Now that we know which are the different ways to instantiate MVC groups we can go back to customizing them.
The simplest way is to pass in new values as part of the arguments map that
mvcGroupInit()
receives, for example
MVCGroup group = application.mvcGroupManager.buildMVCGroup('foo', [key: 'foo'])
However is you wish to use the special config
key that every MVC group configuratio
may have then you must instantiate the group in the following way
MVCGroupConfiguration configuration = application.mvcGroupManager
.cloneMVCConfiguration('foo', [key: 'someValue'])
MVCGroup group = configuration.create()
Note that you can still send custom arguments to the create()
method.
4.2.1. Configuring MVC groups
The following options are available to all MVC groups as long as you use the config
key.
Disabling Lifecycle Events
Every MVC group triggers a few events during the span of its lifetime. These events will be sent to the event bus even if no component is interested in handling them. There may be times when you don’t want these events to be placed in the event bus in order to speed up group creation/destruction. Use the following configuration to gain this effect:
mvcGroups {
// MVC Group for "sample"
'sample' {
model = 'sample.SampleModel'
view = 'sample.SampleView'
controller = 'sample.SampleController'
config {
events {
lifecycle = false
}
}
}
}
The following events will be disabled with this setting:
Disabling Instantiation Events
The Griffon runtime will trigger an event for every artifact it manages. As with the previous events this one will be sent to the event bus even if no component handles it. Skipping publication of this event may result in a slight increase of speed during group instantiation. Use the following configuration to gain this effect:
mvcGroups {
// MVC Group for "sample"
'sample' {
model = 'sample.SampleModel'
view = 'sample.SampleView'
controller = 'sample.SampleController'
config {
events {
instantiation = false
}
}
}
}
The following events will be disabled with this setting:
Disabling Destruction Events
This is the counterpart of the NewInstance
event. Skipping publication of this event
may result in a slight increase of speed when a group or any artifact instance is destroyed.
Use the following configuration to gain this effect:
mvcGroups {
// MVC Group for "sample"
'sample' {
model = 'sample.SampleModel'
view = 'sample.SampleView'
controller = 'sample.SampleController'
config {
events {
destruction = false
}
}
}
}
The following events will be disabled with this setting:
Disabling Controllers as Application Event Listeners
Controllers are registered as application event handlers by default when a group is instantiated. This makes it very convenient to have them react to events placed in the application’s event bus. However you may want to avoid this automatic registration altogether, as it can lead to performance improvements. You can disable this feature with the following configuration:
mvcGroups {
// MVC Group for "sample"
'sample' {
model = 'sample.SampleModel'
view = 'sample.SampleView'
controller = 'sample.SampleController'
config {
events {
listener = false
}
}
}
}
You can still manually register a controller as an application event handler at any time,
with the caveat that it’s now your responsibility to unregister it when the time is
appropriate, most typically during the group’s destroy sequence when mvcGroupDestroy()
is invoked.
4.3. The @MVCAware Transformation
Any component may gain the ability to create and destroy MVC groups through a MVCGroupManager
instance. You only need annotate the class with @griffon.transform.MVCAware
and it will automatically gain all methods exposed by MVCHandler
.
This feature is just a shortcut to avoid reaching for the application instance from objects that do not hold a reference to it.
Here’s an example of a custom bean that’s able to work with MVC groups
@griffon.transform.MVCAware
class Bean {
}
This class can be used in the following way
class SampleService {
@Inject Bean bean
void buildSecondary(String groupName) {
def (m, v, c) = bean.createMVCGroup(groupName)
// do something with m, v and c
}
}
5. Models
This chapter describe models and all binding options.
Models are very simple in nature. Their responsibility is to hold data that can be used by both Controller and View to communicate with each other. In other words, Models are not equivalent to domain classes.
5.1. Swing Binding
Binding in Griffon is achieved by leveraging Java Beans' java.beans.PropertyChangeEvent
and their related classes, thus binding will work with any class that fires this type of
event, regardless of its usage of @griffon.transform.Observable
or not.
5.3. The @Observable AST Transformation
The @griffon.transform.Observable
transformation will inject the behavior of Observable
into the annotated class. It basically injects an instance of java.beans.PropertyChangeSupport
and all methods required to make the model an observable class. It will also make sure that
a java.beans.PropertyChangeEvent
is fired for each observable property whenever said
property changes value.
The following is a list of all methods added by @griffon.transform.Observable
-
void addPropertyChangeListener(PropertyChangeListener listener)
-
void addPropertyChangeListener(String propertyName, PropertyChangeListener listener)
-
void removePropertyChangeListener(PropertyChangeListener listener)
-
void removePropertyChangeListener(String propertyName, PropertyChangeListener listener)
-
PropertyChangeListener[] getPropertyChangeListeners()
-
PropertyChangeListener[] getPropertyChangeListeners(String propertyName)
-
void firePropertyChange(String propertyName, Object oldValue, Object newValue)
5.4. The @Vetoable AST Transformation
The @griffon.transform.Vetoable
transformation will inject the behavior of Vetoable
into the annotated class. It basically injects an instance of java.beans.VetoableChangeSupport
and all methods required to make the model a vetoable class. It will also make sure that
a java.beans.PropertyChangeEvent
is fired for each vetoable property whenever said
property changes value.
The following is a list of all methods added by @griffon.transform.Vetoable
-
void addVetoableChangeListener(VetoableChangeListener listener)
-
void addVetoableChangeListener(String propertyName, VetoableChangeListener listener)
-
void removeVetoableChangeListener(VetoableChangeListener listener)
-
void removeVetoableChangeListener(String propertyName, VetoableChangeListener listener)
-
VetoableChangeListener[] getVetoableChangeListeners()
-
VetoableChangeListener[] getVetoableChangeListeners(String propertyName)
-
void fireVetoableChange(String propertyName, Object oldValue, Object newValue)
5.5. The @PropertyListener AST Transformation
The @griffon.transform.PropertyListener
helps you to register PropertyChangeListener
s
without so much effort. The following code
import griffon.transform.PropertyListener
import griffon.transform.Observable
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonModel)
@PropertyListener(snoopAll)
class SampleModel {
def controller
@Observable String name
@Observable
@PropertyListener({controller.someAction(it)})
String lastname
def snoopAll = { evt -> ... }
}
is equivalent to this one
import java.beans.PropeetyChangeListener
import griffon.transform.Observable
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonModel)
@PropertyListener(snoopAll)
class SampleModel {
def controller
@Observable String name
@Observable String lastname
def snoopAll = { evt -> ... }
SampleModel() {
addPropertyChangeListener(snoopAll as PropertyChangeListener)
addPropertyChangeListener('lastname', {
controller.someAction(it)
} as PropertyChangeListener)
}
}
@griffon.transform.PropertyListener
accepts the following values
-
in-place definition of a closure
-
reference of a closure property defined in the same class
-
a List of any of the previous two
6. Controllers
Controllers are the entry point for your application’s logic. Each controller has
access to their model
and view
instances from their respective MVC group.
Controller actions are defined as public methods on a controller class, for example in Groovy you’d write
package sample
import griffon.core.artifact.GriffonController
@griffon.metadata.ArtifactProviderFor(GriffonController)
class SampleController {
def model
void click() {
model.clickCount++
}
}
The corresponding code for Java 8 would be
package sample;
import griffon.core.artifact.GriffonController;
@griffon.metadata.ArtifactProviderFor(GriffonController.class)
public class SampleController {
private SampleModel model;
public void setModel(SampleModel model) {
this.model = model;
}
public void click() {
runInsideUIAsync(() -> {
model.setClickCount(model.getClickCount() + 1);
});
}
}
Actions must follow these rules in order to be considered as such:
-
must have public visibility modifier.
-
name does not match an event handler, i.e, it does not begin with
on
. -
must pass
GriffonClassUtils.isPlainMethod()
. -
must have
void
as return type.
The application’s ActionManager
will automatically configure an Action
instance for each matching controller action. These Action
instances can be later used
within Views to link them to UI components. The following example shows a Swing View
making use of the configured clickAction
from SampleController
package sample
import griffon.core.artifact.GriffonView
@griffon.metadata.ArtifactProviderFor(GriffonView)
class SampleView {
FactoryBuilderSupport builder
SampleModel model
void initUI() {
builder.with {
application(title: 'Clicker', pack: true)) {
button(clickAction, label: bind { model.clickCount })
}
}
}
}
Controllers can perform other tasks too:
-
listen to application events.
-
react to MVC initialization/destruction via a pair of methods (
mvcGroupInit()
,mvcGroupDestroy()
). -
hold references to services.
-
participate in dependency injection (members must be annotated with
@javax.inject.Inject
).
6.1. Actions and Threads
A key aspect that you must always keep in mind is proper threading. Often times controller actions will be bound in response to an event driven by the UI. Those actions will usually be invoked in the same thread that triggered the event, which would be the UI thread. When that happens you must make sure that the executed code is short and that it quickly returns control to the UI thread. Failure to do so may result in unresponsive applications.
The following example is the typical usage one finds out there
package sample
import groovy.sql.Sql
import java.awt.event.ActionEvent
import griffon.core.artifact.GriffonController
@griffon.metadata.ArtifactProviderFor(GriffonController)
class BadController {
def model
void badAction(ActionEvent evt = null) {
def sql = Sql.newInstance(
app.config.datasource.url,
model.username,
model.password,
app.config.datasource.driver
)
model.products.clear()
sql.eachRow("select * from products") { product ->
model.products << [product.id, product.name, product.price]
}
sql.close()
}
}
What’s wrong with this code? It’s very likely that this action is triggered by clicking on a button, in which case its body will be executed inside the UI thread. This means the database query will be executed on the UI thread too. The model is also updated, once could assume the model is bound to an UI component. This update should happen inside the UI thread, but clearly that’s not what’s happening here.
In order to simplify things the Griffon runtime (via the ActionManager
) assumes
by default that all actions will be invoked outside of the UI thread. This solves the
first problem, that of realizing a database operation on the wrong thread. The second
problem, updating the model, can be solved in the following manner
package sample
import groovy.sql.Sql
import java.awt.event.ActionEvent
import griffon.core.artifact.GriffonController
@griffon.metadata.ArtifactProviderFor(GriffonController)
class GoodController {
def model
void goodAction(ActionEvent evt = null) { (1)
def sql = Sql.newInstance(
app.config.datasource.url,
model.username,
model.password,
app.config.datasource.driver
)
try {
List results = []
sql.eachRow("select * from products") { product ->
results << [product.id, product.name, product.price]
}
runInsideUIAsync { (2)
model.products.clear()
model.products.addAll(results)
}
} finally {
sql.close()
}
}
}
1 | Executed outside the UI thread |
2 | Go back inside the UI thread |
There are other options at your disposal to make sure the code behaves properly according to the specific threading rules of a particular UI toolkit. These options are covered in the threading chapter.
6.2. The ActionManager
Controller actions may automatically be wrapped and exposed as toolkit specific actions; this greatly simplifies how actions can be configured based on i18n concerns.
At the heart of this feature lies the ActionManager
. This component is responsible
for instantiating, configuring and keeping references to all actions per controller.
It will automatically harvest all action candidates from a Controller once it has been
instantiated. Each action has all of its properties configured following this strategy:
-
match
<controller.class.name>
.action.<action.name>
.<key>
-
match application.action.
<action.name>
.<key>
<action.name>
should be properly capitalized. In other words, you can configure action
properties specifically per Controller or application wide. Available keys are
Key | Type | Default Value |
---|---|---|
name |
String |
Natural name minus the |
accelerator |
String |
undefined |
long_description |
String |
undefined |
short_description |
String |
undefined |
mnemonic |
String |
undefined |
small_icon |
String |
undefined |
large_icon |
String |
undefined |
enabled |
boolean |
true |
selected |
boolean |
false |
Key | Type | Default Value |
---|---|---|
name |
String |
Natural name minus the |
accelerator |
String |
undefined |
long_description |
String |
undefined |
short_description |
String |
undefined |
mnemonic |
String |
undefined |
small_icon |
String |
undefined |
large_icon |
String |
undefined |
enabled |
boolean |
true |
selected |
boolean |
false |
Key | Type | Default Value |
---|---|---|
name |
String |
Natural name minus the |
description |
String |
undefined |
enabled |
boolean |
true |
Key | Type | Default Value |
---|---|---|
name |
String |
Natural name minus the |
Icon keys should point to an URL available in the classpath.
Values must be placed in resources files following the internationalization guidelines.
6.2.1. Configuration Examples
The following Controller defines 2 actions, one of them uses the Action
suffix because its name clashes
with a known Java keyword.
package sample
import java.awt.event.ActionEvent
import griffon.core.artifact.GriffonController
@griffon.metadata.ArtifactProviderFor(GriffonController)
class SampleController {
void close(ActionEvent evt) { ... }
void newAction(ActionEvent evt) { ... }
}
The ActionManager
will generate and configure the following actions:
-
newAction
-
closeAction
The following keys are expected to be available in the application’s i18n resources (i.e. griffon-app/i18n/messages.properties
)
sample.SampleController.action.New.name = New
sample.SampleController.action.Open.name = Open
sample.SampleController.action.Close.name = Close
sample.SampleController.action.Delete.name = Delete
# additional keys per action elided
In the case that you’d like the close action to be customized for all controllers, say using
the Spanish language, then you’ll have to provide a file named griffon-app/i18n/messages_es.properties
with the following keys
application.action.Close.name = Cerrar
Make sure to remove any controller specific keys when reaching for application wide configuration.
6.3. Action Interceptors
ActionInterceptor
s open a new set of possibilities by allowing developers
and addon authors to define code that should be executed before and after
any controller action is invoked by the framework. For example, you may want to protect
the execution of a particular action given specific permissions; the shiro
plugin uses
annotations that are handled by an ActionInterceptor
, like this
import griffon.core.artifact.GriffonController
import griffon.metadata.ArtifactProviderFor
import griffon.plugins.shiro.annotation.*
@ArtifactProviderFor(GriffonController)
@RequiresAuthentication
class PrinterController {
@RequiresPermission('printer:print')
void print () { ... }
@RequiresRoles('administrator')
void configure() { ... }
}
The scaffolding
plugin on the other hand modifies the arguments sent to the action.
Take the following snippet for example
import griffon.core.artifact.GriffonController
import griffon.metadata.ArtifactProviderFor
import griffon.plugins.shiro.annotation.*
import org.apache.shiro.authc.UsernamePasswordToken
impprt org.apache.shiro.subject.Subject
import javax.swing.JOptionPane
import javax.inject.Inject
@ArtifactProviderFor(GriffonController)
class StrutsController {
@Inject
private Subject subject
@RequiresGuest
void login(LoginCommandObject cmd) {
try {
subject.login(new UsernamePasswordToken(cmd.username, cmd.password))
} catch(Exception e) {
JOptionPane.showMessageDialog(
app.windowManager.findWindow('mainWindow'),
'Invalid username and/or password',
'Security Failure', JOptionPane.ERROR_MESSAGE)
}
}
@RequiresAuthentication
void logout() {
subject.logout()
}
}
Note that the login
action requires an instance of LoginCommandObject
. The scaffolding
plugin is aware of this fact; it will create an instance of said class, wire up an scaffolded
view in a dialog and present it to the user. The LoginCommandObject
instance will be set
as the action’s arguments if it validates successfully, otherwise action execution is aborted.
6.3.1. Implementing an Action Interceptor
Action interceptors must implement the ActionInterceptor
interface. There’s a
handy base class (org.codehaus.griffon.runtime.core.controller.AbstractActionInterceptor
)
that provides sensible defaults. Say you’d want to know how much time it took for an action
to be executed, also if an exception occurred during its execution. This interceptor could
be implemented as follows
package com.acme
import griffon.core.artifact.GriffonController
import griffon.core.controller.ActionExecutionStatus
import org.codehaus.griffon.runtime.core.controller.AbstractActionInterceptor
import javax.inject.Named
@Named('tracer')
class TracerActionInterceptor extends AbstractActionInterceptor {
private final Map<String, Long> TIMES = [:]
Object[] before(GriffonController controller, String actionName, Object[] args) {
TIMES[qualifyActionName(controller, actionName)] = System.currentTimeMillis()
return super.before(controller, actionName, args)
}
void after(ActionExecutionStatus status, GriffonController controller, String actionName, Object[] args) {
String qualifiedActionName = qualifyActionName(controller, actionName)
long time = System.currentTimeMillis() - TIMES[qualifiedActionName]
println("Action ${qualifiedActionName} took ${time} ms [${status}]")
}
}
The ActionInterceptor
interface defines a handful of methods that are invoked
by the ActionManager
at very specific points during the lifetime and execution
of controller actions.
- void configure(GriffonController controller, String actionName, Method method)
-
The
configure()
method is called during the configuration phase, when theActionManager
creates the actions. This method is called once in the lifetime of an action. - Object[] before(GriffonController controller, String actionName, Object[] args)
-
The
before()
method is executed every time an action is about to be invoked. This method is responsible for adjusting the arguments (if needed) or aborting the action execution altogether. Any exception thrown by an interceptor in this method will halt action execution however onlyAbortActionExecution
is interpreted as a graceful abort. - boolean exception(Exception exception, GriffonController controller, String actionName, Object[] args)
-
The
exception()
method is invoked only when an exception occurred during the action’s execution. Implementors must returntrue
if the exception was handled successfully. The exception will be rethrown by theActionManager
if no interceptor handled the exception. This happens as the last step of the action interception procedure. - void after(ActionExecutionStatus status, GriffonController controller, String actionName, Object[] args)
-
The
after()
method is called after an action has been executed. Any exceptions occurred during the action’s execution should have been handled byexception()
. Thestatus
argument specifies if the action was successfully executed (OK
), if it was aborted by an interceptor (ABORTERD
) or if an exception occurred during its execution (EXCEPTION
).
Action interceptors can participate in Dependency Injection.
6.3.2. Configuration
Action Interceptors must be registered within a Module
in order to be picked
up by the ActionManager
. The following example shows how the previous TracerActionInterceptor
can be registered in a Module
package com.acme
import griffon.core.injection.Module
import griffon.core.controller.ActionInterceptor
import org.codehaus.griffon.runtime.core.injection.AbstractModule
import org.kordamp.jipsy.ServiceProviderFor
import javax.inject.Named
@ServiceProviderFor(Module)
@Named('application')
public class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(ActionInterceptor)
.to(TracerActionInterceptor)
.asSingleton()
}
}
An Interceptor may define a dependency on another interceptor; use the @griffon.inject.DependsOn
annotation to express the relationship.
It’s also possible to globally override the order of execution of interceptors, or
define and order when interceptors are orthogonal. Take for example the security
interceptor provided by the shiro
plugin and the scaffolding
interceptor provided by
scaffolding
plugin. These interceptors know nothing about each other however security
should be called before scaffolding
. This can be accomplished by adding the following
snippet to Config.groovy
griffon.controller.action.interceptor.order = ['security', 'scaffolding']
7. Services
Services are responsible for the application logic that does not belong to a single
controller. They are meant to be treated as singletons, injected to MVC members by
following a naming convention. Services must be located inside the griffon-app/services
Let’s say you want to create a Math service. A trivial implementation of an addition
operation performed by the MathService
would look like the following snippet
package sample
import griffon.core.artifact.GriffonService
@griffon.metadata.ArtifactProviderFor(GriffonService)
class MathService {
def addition(a, b) { a + b }
}
Using this service from a Controller is a straight forward task, you just have to
define an injection point and annotated it with @javax.inject.Inject
package sample
import griffon.core.artifact.GriffonController
@griffon.metadata.ArtifactProviderFor(GriffonController)
class SampleController
SampleModel model
@javax.inject.Inject
private MathService mathService
void calculate(evt = null) {
model.result = mathService.addition model.a, model.b
}
}
Given that services are inherently treated as singletons they are also automatically
registered as application event listeners. Be aware that services will be instantiated
lazily which means that some events might not reach a particular service if it has not
been instantiated by the framework by the time of event publication. It’s also discouraged
to use Groovy’s @Singleton
annotation on a Service class as it will cause trouble with
the automatic singleton management Griffon has in place.
8. Views
8.1. The WindowManager
Although the following API refers directly to Swing
in all examples it’s possible to use
the WindowManager
with other toolkits such as JavaFX
, Pivot
and Lanterna
as
there are specific WindowManager
implementations plus helper classes for those
UI toolkits too.
The WindowManager
class is responsible for keeping track of all the windows
managed by the application. It also controls how these windows are displayed (via a
pair of methods: show
, hide
). WindowManager
relies on an instance of
WindowDisplayHandler
to actually show or hide a window. The default implementation
simple shows and hide windows directly, however you can change this behavior by setting
a different implementation of WindowDisplayHandler
on the application instance.
8.1.1. WindowManager DSL
This configuration can be set in griffon-app/conf/Config.groovy
file, here is how it looks
windowManager {
myWindowName = [
show: {name, window -> ... },
hide: {name, window -> ... }
]
myOtherWindowName = [
show: {name, window -> ... }
]
}
The name of each entry must match the value of the Window’s name:
property (if supported)
or the name used to register the Window with the WindowManager
. Each entry may
have any the following options
- show
-
Used to show the window to the screen. It must be a closure that takes two parameters: the name of the window and the window to be displayed.
- hide
-
Used to hide the window from the screen. It must be a closure that takes two parameters: the name of the window and the window to be hidden.
- handler
-
A custom
WindowDisplayHandler
instance.
You must use CallableWithArgs instead of closures if using the Java version
of the Config file.
|
The first two options have priority over the third one. If one is missing then the
WindowManager
will invoke the default behavior. There is one last option
that can be used to override the default behavior provided to all windows
windowManager {
defaultHandler = new MyCustomWindowDisplayHandler()
}
You can go a bit further by specifying a global show or hide behavior as shown in the following example
windowManager {
defaultShow: {name, window -> ... },
myWindowName = [
show: {name, window -> ... },
hide: {name, window -> ... }
]
myOtherWindowName = [
show: {name, window -> ... }
]
}
8.1.2. Starting Window
By default the WindowManager
picks the first available window from the managed
windows list to be the starting window. However, this behavior can be configured,
by means of the WindowManager DSL. Simply specify a value for windowManager.startingWindow
,
like this
windowManager {
startingWindow = 'primary'
}
This configuration flag accepts two types of values:
-
a String that defines the name of the Window. You must make sure the Window has a matching name property or was attached to the
WindowManager
with the same name -
a Number that defines the index of the Window in the list of managed windows.
If no match is found then the default behavior will be executed.
8.1.3. Custom WindowDisplayHandlers
The following example shows how you can center on screen all managed windows
package sample
import org.codehaus.griffon.runtime.swing.DefaultSwingWindowDisplayHandler
import javax.annotation.Nonnull
import java.awt.Window
import static griffon.swing.support.SwingUtils.centerOnScreen
class CenteringWindowDisplayHandler extends DefaultSwingWindowDisplayHandler {
@Override
void show(@Nonnull String name, @Nonnull Window window) {
centerOnScreen(window)
super.show(name, window)
}
}
You can register CenteringWindowDisplayHandler
using the WindowManager DSL. Alternatively
you may use a Module to register the class/instance.
package sample
import griffon.core.injection.Module
import griffon.inject.DependsOn
import griffon.swing.SwingWindowDisplayHandler
import org.codehaus.griffon.runtime.core.injection.AbstractModule
import org.kordamp.jipsy.ServiceProviderFor
import static griffon.util.AnnotationUtils.named
@DependsOn('swing')
@ServiceProviderFor(Module)
class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(SwingWindowDisplayHandler)
.withClassifier(named('defaultWindowDisplayHandler'))
.to(CenteringWindowDisplayHandler)
.asSingleton()
}
}
This example is equivalent to defining a WindowDisplayHandler
for all windows.
You may target specific windows, by define multiple bindings, making sure that the name
of the classifier matches the window name. Notice the explicit dependency on the swing
module.
If this dependency is left out it’s very likely that the WindowManager
will fail
to pick the correct WindowDisplayHandler
.
9. Threading
Building a well behaved multi-threaded desktop application has been a hard task for many years, however it does not have to be that way anymore. The following sections explain the threading facilities exposed by the Griffon framework.
9.1. Synchronous Calls
Synchronous calls inside the UI thread are made by invoking the runInsideUISync
method.
This method results in the same behavior as calling SwingUtilities.invokeAndWait()
when
using Swing.
package sample
import java.awt.event.ActionEvent
import griffon.core.artifact.GriffonController
@griffon.metadata.ArtifactProviderFor(GriffonController)
class SampleController {
def model
void work(evt = null) {
// will be invoked outside of the UI thread by default
def value = model.value
// do some calculations
runInsideUISync {
// back inside the UI thread
model.result = ...
}
}
}
9.2. Asynchronous Calls
Similarly to synchronous calls, asynchronous calls inside the UI thread are made by
invoking the runInsideUIAsync
method. This method results in the same behavior as
calling SwingUtilities.invokeLater()
when using Swing.
package sample
import java.awt.event.ActionEvent
import griffon.core.artifact.GriffonController
@griffon.metadata.ArtifactProviderFor(GriffonController)
class SampleController {
def model
void work(evt = null) {
// will be invoked outside of the UI thread by default
def value = model.value
// do some calculations
runInsideUIAsync {
// back inside the UI Thread
model.result = ...
}
}
}
9.3. Outside Calls
Making sure a block of code is executed outside the UI thread is made by invoking
the execOutsideUI
method. This method is smart enough to figure out if the unit
of work is already outside of the UI thread; otherwise it instructs the Griffon
runtime to runt he unit in a different thread. This is usually performed by a
helper java.util.concurrent.ExecutorService
.
package sample
import java.awt.event.ActionEvent
import griffon.core.artifact.GriffonController
@griffon.metadata.ArtifactProviderFor(GriffonController)
class SampleController {
def model
void work(evt = null) {
// will be invoked outside of the UI thread by default
def value = model.value
// do some calculations
runInsideUIAsync {
// back inside the UI thread
model.result = ...
runOutsideUI {
// do more calculations
}
}
}
}
9.4. Additional Threading Methods
There are two additional methods that complement the generic threading facilities that Griffon exposes to the application and its artifacts
- isUIThread()
-
Returns
true
if the current thread is the UI thread,false
otherwise. Functionally equivalent to callingSwingUtilities.isEventDispatchThread()
in Swing. - runFuture(ExecutorService s, Callable c)
-
schedules a callable on the target
ExecutorService
. The executor service can be left unspecified, if so a default Thread pool executor will be used.
9.5. The @Threading Annotation
The @griffon.transform.Threading
annotation can be used to alter the default behavior of
executing a controller action outside of the UI thread. There are 4 possible values
that can be specified for this annotation
- INSIDE_UITHREAD_SYNC
-
Executes the code in a synchronous call inside the UI thread. Equivalent to wrapping the code with
runInsideUISync
. - INSIDE_UITHREAD_ASYNC
-
Executes the code in an asynchronous call inside the UI thread. Equivalent to wrapping the code with
runInsideUIAsync
. - OUTSIDE_UITHREAD
-
Executes the code outside of the UI thread. Equivalent to wrapping the code with
runOutsideUI
. - SKIP
-
Executes the code in the same thread as the invoker, whichever it may be.
This annotation can be usend as an AST transformation on any other component that’s not a controller. Any component may gain the ability to execute code in a particular thread, following the selected UI toolkit’s execution rules.
Here’s an example of a custom component that’s able to call its methods in different threads
package sample
import griffon.transform.Threading
class Sample {
@Threading
void doStuff() {
// executed outside of the UI thread
}
@Threading(Threading.Policy.INSIDE_UITHREAD_SYNC)
void moreStuff() {
// executed synchronously inside the UI thread
}
}
You must annotate a method with @griffon.transform.Threading
. Annotated methods must conform to these rules
-
must be public.
-
name does not match an event handler.
-
must pass
GriffonClassUtils.isPlainMethod()
. -
must have @void@ as return type.
9.6. The @ThreadingAware AST Transformation
Any component may gain the ability to execute code in a particular thread, following
the selected UI toolkit’s execution rules. It injects the behavior of ThreadingHandler
into the annotated class.
This feature is just a shortcut to avoid reaching for the UIThreadManager
instance
from objects that do not hold a reference to it.
Here’s an example of a custom component that’s able to call its methods in different threads
package sample
@griffon.transform.ThreadingAware
class Sample {
void doStuff() {
runOutsideUIThread {
// executed outside of the UI thread
}
}
void moreStuff() {
runInsideUIAsync {
// executed asynchronously inside the UI thread
}
}
}
10. Events
Applications have the ability to publish events from time to time to communicate that something of interest has happened at runtime. Events will be triggered by the application during each of its life cycle phases, also when MVC groups are created and destroyed.
All application event handlers are guaranteed to be called in the same thread that originated the event. |
10.1. Publishing Events
Any instance that obtains a reference to an EventRouter
can publish events.
GriffonApplication
exposes the application wide EventRouter
via a read-only
property. You may use Dependency Injection to inject an EventRouter
to any class too.
Publishing an event can be done synchronously on the current thread, asynchronously to the current thread, or asynchronously relative to the UI thread. For example, the following snippet will trigger an event that will be handled in the same thread, which could be the UI thread itself
application.eventRouter.publish('MyEventName', ['arg0', 'arg1'])
Whereas the following snippet guarantees that all event handlers that are interested in
an event of type MyEventName
will be called outside of the UI thread
application.eventRouter.publishOutsideUI('MyEventName', ['arg0', 'arg1'])
Finally, if you’d want event notification to be handed in a thread that is not the current one (regardless if the current one is the UI thread or not) then use the following method
application.eventRouter.publishAsync('MyEventName', ['arg0', 'arg1'])
Alternatively, you may specify an instance of a subclass of Event
as the sole
argument to any of these methods. The event instance will be the single argument sent
to the event handlers when the event
methods are invoked in this way.
There may be times when event publishing must be stopped for a while. If that’s the case then you can instruct the application to stop delivering events by invoking the following code
application.eventRouter.eventPublishingEnabled = false
Any events sent through the application’s event bus will be discarded after that call; there’s no way to get them back or replay them. When it’s time to enable the event bus again simply call
application.eventRouter.eventPublishingEnabled = true
10.2. Consuming events
Any artifact or class that abides to the following conventions can be registered as an application listener, those conventions are:
-
it is a Map, a
CallableWithArgs
or an Object. -
in the case of a Map, each key maps to
<EventName>
, the value must be a CallableWithArgs. -
in the case of object, public methods whose name matches
on<EventName>
will be used as event handlers. -
Objects and maps can be registered/unregistered by calling
addApplicationListener()
/removeApplicationListener()
on theEventRouter
instance. -
CallableWithArgs event handlers must be registered with an overloaded version of
addApplicationListener()
/removeApplicationListener()
that takes<EventName>
as the first parameter, and the callable itself as the second parameter.
There is a global, per-application event handler that can be registered. If you want
to take advantage of this feature you must define a class that implements the
EventHandler
interface. This class must be registered with a Module
.
Lastly both Controller and Service instances are automatically registered as application
event listeners.
10.2.1. Examples
These are some examples of event handlers:
Display a message right before default MVC groups are instantiated
package com.acme
import griffon.core.GriffonApplication
import griffon.core.event.EventHandler
class ApplicationEventHandler implements EventHandler {
void onBootstrapEnd(GriffonApplication application) {
println """
Application configuration has finished loading.
MVC Groups will be initialized now.
""".stripIndent(12)
}
}
package com.acme
import griffon.core.event.EventHandler
import griffon.core.injection.Module
import org.codehaus.griffon.runtime.core.injection.AbstractModule
import org.kordamp.jipsy.ServiceProviderFor
@ServiceProviderFor(Module)
class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(EventHandler)
.to(ApplicationEventHandler)
.asSingleton()
}
}
Print the name of the application plus a short message when the application is about to shut down.
class MyController {
void onShutdownStart(application)
println "${application.configuration['application.title']} is shutting down"
}
}
Print a message every time the event "Foo" is published
class MyController {
void mvcGroupInit(Map<String, Object> args) {
application.eventRouter.addEventListener([
Foo: { println 'got foo!' } as CallableWithArgs
])
}
void fooAction() {
// do something
application.eventRouter.publish('Foo')
}
}
An alternative to the previous example using a CallableWithArgs
event handler
class MyController {
void mvcGroupInit(Map<String, Object> args) {
application.eventRouter.addEventListener('Foo',
{ println 'got foo!' } as CallableWithArgs
])
}
void fooAction() {
// do something
application.eventRouter.publish('Foo')
}
}
An alternative to the previous example using a custom event class
class MyController {
void mvcGroupInit(Map<String, Object> args) {
application.eventRouter.addListener(Foo) {
e -> assert e instanceof Foo
}
}
void fooAction() {
// do something
application.eventRouter.publish(new MyController.Foo(this))
}
static class Foo extends griffon.core.Event {
Foo(Object source) { super(source) }
}
}
10.3. Application Events
The following events will be triggered by the application when dealing with artifacts
- NewInstance(Class klass, Object instance)
-
When a new artifact is created.
- DestroyInstance(Class klass, Object instance)
-
When an artifact instance is destroyed.
- LoadAddonsStart(GriffonApplication application)
-
Before any addons are initialized, during the Initialize phase.
- LoadAddonsEnd(GriffonApplication application, Map<String, GriffonAddon> addons)
-
After all addons have been initialized, during the Initialize phase.
- LoadAddonStart(String name, GriffonAddon addon, GriffonApplication application)
-
Before an addon is initialized, during the Initialize phase.
- LoadAddonEnd(String name, GriffonAddon addon, GriffonApplication application)
-
After an addon has been initialized, during the Initialize phase.
These events will be triggered when dealing with MVC groups
- InitializeMVCGroup(MVCGroupConfiguration configuration, MVCGroup group)
-
When a new MVC group is initialized.
- CreateMVCGroup(MVCGroup group)
-
When a new MVC group is created.
- DestroyMVCGroup(MVCGroup group)
-
When an MVC group is destroyed.
10.4. Lifecycle Events
The following events will be triggered by the application during each one of its phases
- BootstrapStart(GriffonApplication application)
-
After logging configuration has been setup, during the Initialize phase.
- BootstrapEnd(GriffonApplication application)
-
At the end of the Initialize phase.
- StartupStart(GriffonApplication application)
-
At the beginning of the Startup phase.
- StartupEnd(GriffonApplication application)
-
At the end of the Startup phase.
- ReadyStart(GriffonApplication application)
-
At the beginning of the Ready phase.
- ReadyEnd(GriffonApplication application)
-
At the end of the ready phase.
- ShutdownRequested(GriffonApplication application)
-
Before the Shutdown begins.
- ShutdownAborted(GriffonApplication application)
-
If a
ShutdownHandler
prevented the application from entering the Shutdown phase. - ShutdownStart(GriffonApplication application)
-
At the beginning of the Shutdown phase.
10.5. Miscellaneous Events
These events will be triggered when a specific condition is reached
- WindowShown(W window)
-
Triggered by the
WindowManager
when a window is shown. - WindowHidden(W window)
-
Triggered by the
WindowManager
when a window is hidden.
10.6. The @EventPublisher AST Transformation
Any component may gain the ability to publish events through an EventRouter
instance. You only need annotate the class with @griffon.transform.EventPublisher
and it will automatically gain all methods exposed by EventPublisher
.
The following example shows a trivial usage of this feature
@griffon.transform.EventPublisher
class Publisher {
void doit(String name) {
publishEvent('arg', [name])
}
void doit() {
publishEvent('empty')
}
}
The application’s event router will be used by default. If you’d like your custom
event publisher to use a private EventRouter
then you must define a binding
for it using a specific name, like this
import griffon.core.injection.Module
import griffon.core.event.EventRouter
import org.kordamp.jipsy.ServiceProviderFor
import org.codehaus.griffon.runtime.core.injection.AbstractModule
import org.codehaus.griffon.runtime.core.event.DefaultEventRouter
import javax.inject.Named
import static griffon.util.AnnotationUtils.named
@ServiceProviderFor(Module)
@Named
class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(EventRouter)
.withClassifier(named('my-private-event-router'))
.to(DefaultEventRouter)
.asSingleton()
}
}
Next specify the named EventRouter
as a parameter on the @griffon.transform.EventPublisher
transformation
@griffon.transform.EventPublisher('my-private-event-router')
class Publisher {
void doit(String name) {
publishEvent('arg', [name])
}
void doit() {
publishEvent('empty')
}
}
11. Internationalization
This chapter describes Internationalization (I18N
) features available to all applications.
11.1. MessageSource
Applications have the ability to resolve internationalizable messages by leveraging
the behavior exposed by MessageSource
. This interface exposes the following methods:
-
String getMessage(String key)
-
String getMessage(String key, Locale locale)
-
String getMessage(String key, Object[] args)
-
String getMessage(String key, Object[] args, Locale locale)
-
String getMessage(String key, List args)
-
String getMessage(String key, List args, Locale locale)
-
String getMessage(String key, Map args)
-
String getMessage(String key, Map args, Locale locale)
-
Object resolveMessageValue(String key, Locale locale)
The first set throws NoSuchMessageException
if a message could not be resolved given
the key sent as argument. The following methods take and additional defaultMessage
parameter that may be used if no configured message is found. If this optional parameter
were to be null then the key
is used as the message; in other words, these methods
never throw NoSuchMessageException
nor return null
unless the passed in key
is null.
-
String getMessage(String key, String defaultMessage)
-
String getMessage(String key, Locale locale, String defaultMessage)
-
String getMessage(String key, Object[] args, String defaultMessage)
-
String getMessage(String key, Object[] args, Locale locale, String defaultMessage)
-
String getMessage(String key, List args, String defaultMessage)
-
String getMessage(String key, List args, Locale locale, String defaultMessage)
-
String getMessage(String key, Map args, String defaultMessage)
-
String getMessage(String key, Map args, Locale locale, String defaultMessage)
The simplest way to resolve a message thus results like this
getApplication().getMessageSource().getMessage('some.key')
The set of methods that take a List
as arguments are meant to be used from Groovy
code whereas those that take an Object[]
are meant for Java code; this leads to
better idiomatic code as the following examples reveal
getApplication().getMessageSource()
.getMessage('groovy.message', ['apples', 'bananas'])
getApplication().getMessageSource()
.getMessage("java.message", new Object[]{"unicorns", "rainbows"});
Of course you may also use List
versions in Java, like this
getApplication().getMessageSource()
.getMessage("hybrid.message", Arrays.asList("bells", "whistles"));
11.1.1. Message Formats
There are three types of message formats supported by default. Additional formats may be supported if the right plugins are installed. Resources may be configured using either properties files or Groovy scripts, please refer to the configuration section.
Standard Format
The first set of message formats are those supported by the JDK’s
MessageFormat
facilities. These formats work with all versions of the getMessage()
method that
take a List
or an Object[]
as arguments. Examples follow. First the messages
stored in a properties file
healthy.proverb = An {0} a day keeps the {1} away
yoda.says = {0} is the path to the dark side. {0} leads to {1}. {1} leads to {2}. {2} leads to suffering.
Then the code used to resolve them
String quote = getApplication().getMessageSource()
.getMessage('healthy.proverb', ['apple', 'doctor'])
assert quote == 'An apple a day keeps the doctor away'
String quote = getApplication().getMessageSource()
.getMessage("yoday.says", new Object[]{"Fear", "Anger", "Hate"});
assertEquals(quote, "Fear is the path to the dark side. Fear leads to Anger. Anger leads to Hate. Hate leads to suffering");
Map Format
The following format is non-standard (i.e, not supported by MessageFormat
) and can
only be resolved by Griffon. This format uses symbols instead of numbers as placeholders
for arguments. Thus the previous messages can be rewritten as follows
healthy.proverb = An {:fruit} a day keeps the {:occupation} away
yoda.says = {:foo} is the path to the dark side. {:foo} leads to {:bar}. {:bar} leads to {:foobar}. {:foobar} leads to suffering.
Which may be resolved in this manner
String quote = getApplication().getMessageSource()
.getMessage('healthy.proverb', [fruit: 'apple', occupation: 'doctor'])
assert quote == 'An apple a day keeps the doctor away
import static griffon.util.CollectionUtils.map;
String quote = getApplication().getMessageSource()
.getMessage("yoday.says", map().e("foo", "Fear")
.e("bar", "Anger")
.e("foobar","Hate"));
assertEquals(quote, "Fear is the path to the dark side. Fear leads to Anger. Anger leads to Hate. Hate leads to suffering");
Groovy format
Groovy scripts have one advantage over properties files as you can embed custom logic that may conditionally resolve a message based on environmental values or generate a message on the fly. In order to accomplish this feat messages must be defined as closures and must return a String value; if they do not then their return value will be translated to a String. The following message uses the value of the current running environment to determine the text of a warning to be displayed on a label
import griffon.util.Environment
warning.label = { args ->
if (Environment.current == Environment.PRODUCTION) {
"The application has encountered an error: $args"
} else {
"Somebody setup us the bomb! $args"
}
}
11.1.2. Reference Keys
There may be times where you would want to have a 2 keys reference the same value,
as if one key were an alias for the other. MessageSource
supports the notion of
referenced keys for this matter. In order to achieve this, the value of the alias
key must define the aliased key with a special format, for example
famous.quote = This is {0}!
hello.world = @[famous.quote]
Resolving those keys results in
assert getApplication()
.getMessageSource()
.getMessage('famous.quote', ['Sparta']) == 'This is Sparta!'
assert getApplication()
.getMessageSource()
.getMessage('hello.world', ['Griffon']) == 'This is Griffon!'
11.2. MessageSource Configuration
Messages may be configured in either properties files or Groovy scripts.
Groovy scripts have precedence over properties files should there be two files that
match the same basename
. The default configured basename
is "messages
", thus
the application will search for the following resources in the classpath.
-
messages.properties
-
messages.groovy
Of course Groovy scripts are only enabled if you add a dependency to the griffon-groovy
module to your project. The default basename
may be changed to some other value,
or additional basenames may be specified too; it’s just a matter of configuring a
Module override
@ServiceProviderFor(Module.class)
@Named("application")
@DependsOn("core")
public class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(MessageSource.class)
.withClassifier(named("applicationMessageSource"))
.toProvider(new MessageSourceProvider("custom_basename"))
.asSingleton();
}
}
Both properties files and Groovy scripts are subject locale aware loading mechanism
described next. For a Locate set to de_CH_Basel
the following resources will be
searched for and loaded
-
messages.properties
-
messages.groovy
-
messages_de.properties
-
messages_de.groovy
-
messages_de_CH.properties
-
messages_de_CH.groovy
-
messages_de_CH_Basel.properties
-
messages_de_CH_Basel.groovy
Properties files and Groovy scripts used for internationalization purposes are usually
placed under griffon-app/i18n
The default messages.properties
file is placed in
this directory upon creating an application using the standard project templates.
11.3. The @MessageSourceAware AST Transformation
Any component may gain the ability to resolve messages through a MessageSource
instance. You only need annotate the class with @griffon.transform.MessageSourceAware
and it will automatically gain all methods exposed by MessageSource
.
This feature is just a shortcut to avoid reaching for the application instance from objects that do not hold a reference to it.
Here’s an example of a custom bean that’s able to resolve messages
@griffon.transform.MessageSourceAware
class Bean {
String name
}
This class can be used in the following way
class SampleService {
@Inject Bean bean
String lookupValues(String arg) {
bean.name = arg
bean.getMessage('some.message.key', [bean.name])
}
}
The application’s MessageSource
will be injected to annotated beans if no name is
specified as an argument to MessageSourceAware
. You may define multiple MessageSource
bindings as long as you qualify them with a distinct name, such as
@ServiceProviderFor(Module.class)
@Named("application")
public class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(MessageSource.class)
.withClassifier(AnnotationUtils.named("foo"))
.toProvider(new MessageSourceProvider("foofile"))
.asSingleton();
bind(MessageSource.class)
.withClassifier(AnnotationUtils.named("bar"))
.toProvider(new MessageSourceProvider("barfile"))
.asSingleton();
}
}
Then make use of any of these bindings like so
@griffon.transform.MessageSourceAware('foo')
class Bean {
String name
}
12. Resource Management
This chapter describes resource management and injection features available to all applications.
12.1. Locating Classpath Resources
Resources can be loaded form the classpath using the standard mechanism provided by the
Java runtime, that is, ask a ClassLoader
instance to load a resource URL
or obtain
an InputStream
that points to the resource.
But the code can get quite verbose, take for example the following view code that locates a text file and displays it on a text component
scrollPane {
textArea(columns: 40, rows: 20,
text: this.class.classLoader.getResource('someTextFile.txt').text)
}
In order to reduce visual clutter, also to provide an abstraction over resource location,
applications rely on ResourceHandler
, which exposes the following contract
-
URL getResourceAsURL(String name)
-
InputStream getResourceAsStream(String name)
-
List<URL> getResources(String name)
-
ClassLoader classloader()
Thus, the previous example can be rewritten in this way
scrollPane {
textArea(columns: 40, rows: 20,
text: application.resourceHandler.getResourceAsURL('someTextFile.txt').text)
}
In the future Griffon may switch to a modularized runtime, this abstraction will shield
you from any problems when the underlying mechanism changes, such as picking the correct
ClassLoader
.
12.2. The @ResourcesAware AST Transformation
Any component may gain the ability to locate resources through a ResourceHandler
instance. You only need annotate the class with @griffon.transform.ResourcesAware
and it will automatically gain all methods exposed by ResourceHandler
.
This feature is just a shortcut to avoid reaching for the application instance from objects that do not hold a reference to it.
Here’s an example of a custom bean that’s able to locate resources
@griffon.transform.ResourcesAware
class Bean {
String name
}
This class can be used in the following way
class SampleService {
@Inject Bean bean
InputStream fetchResource(String arg) {
bean.name = arg
bean.getResourceAsStream(bean.name)
}
}
12.3. ResourceResolver
Applications have the ability to resolve internationalizable messages by leveraging
the behavior exposed by ResourceResolver
. This interface exposes the following methods:
-
Object resolveResource(String key)
-
Object resolveResource(String key, Locale locale)
-
Object resolveResource(String key, Object[] args)
-
Object resolveResource(String key, Object[] args, Locale locale)
-
Object resolveResource(String key, List args)
-
Object resolveResource(String key, List args, Locale locale)
-
Object resolveResource(String key, Map args)
-
Object resolveResource(String key, Map args, Locale locale)
-
Object resolveResorceValue(String key, Locale locale)
The first set throws NoSuchResourceException
if a message could not be resolved given
the key sent as argument. The following methods take and additional defaultValue
parameter that may be used if no configured resource is found. If this optional parameter
were to be null then the key
is used as the literal value of the resource; in other words,
these methods never throw NoSuchResourceException
nor return null
unless the passed
in key
is null.
-
Object resolveResource(String key, Object defaultValue)
-
Object resolveResource(String key, Locale locale, Object defaultValue)
-
Object resolveResource(String key, Object[] args, Object defaultValue)
-
Object resolveResource(String key, Object[] args, Locale locale, Object defaultValue)
-
Object resolveResource(String key, List args, Object defaultValue)
-
Object resolveResource(String key, List args, Locale locale, Object defaultValue)
-
Object resolveResource(String key, Map args, Object defaultValue)
-
Object resolveResource(String key, Map args, Locale locale, Object defaultValue)
The simplest way to resolve a message thus results like this
getApplication().getResourceResolver().resolveResource('menu.icon')
The set of methods that take a List
as arguments are meant to be used from Groovy
code whereas those that take an Object[]
are meant for Java code; this leads to
better idiomatic code as the following examples reveal
getApplication().getResourceResolver()
.resolveResource('groovy.icon.resource', ['small']))
getApplication().getResourceResolver()
.resolveResource("java.icon.resource", new Object[]{"large"});
Of course you may also use List
versions in Java, like this
getApplication().getResourceResolver()
.resolveResource("hybrid.icon.resource", Arrays.asList("medium"));
12.3.1. Message Formats
There are three types of resource formats supported by default. Additional formats may be supported if the right plugins are installed. Resources may be configured using either properties files or Groovy scripts, please refer to the configuration section.
Standard Format
The first set of resource formats are those supported by the JDK’s
MessageFormat
facilities. These formats work with all versions of the resolveResource()
method that
take a List
or an Object[]
as arguments. Examples follow. First the resource
definitions stored in a properties file
menu.icon = /img/icons/menu-{0}.png
Assuming there are three icon files stored at griffon-app/resources/img/icons
whose
filenames are menu-small.png
, menu-medium.png
and menu-large.png
, a component may
resolve any of them with
Object icon = getApplication().getResourceResolver()
.resolveResource('menu.icon', ['large'])
Map Format
The following format is non-standard (i.e, not supported by MessageFormat
) and can
only be resolved by Griffon. This format uses symbols instead of numbers as placeholders
for arguments. Thus the previous messages can be rewritten as follows
menu.icon = /img/icons/menu-{:size}.png
Which may be resolved in this manner
Object icon = getApplication().getResourceResolver()
.resolveResource('menu.icon', [size: 'large'])
Groovy format
Groovy scripts have one advantage over properties files as you can embed custom logic that may conditionally resolve a resource based on environmental values or generate a message on the fly. In order to accomplish this feat resources must be defined as closures. The following message uses the value of the current running environment to determine the text of a warning to be displayed on a label
import java.awt.Rectangle
direct.instance = new Rectangle(10i, 20i, 30i, 40i)
computed.instance = { x, y, w, h ->
new Rectangle(x, y, w, h)
}
12.3.2. Type Conversion
Note that the return value of resolveResource
is marked as Object
but you’ll get
a String
from the first two formats. You’ll have to rely on property editors
in order to transform the value into the correct type. Injected resources
are automatically transformed to the expected type.
Here’s how it can be done
import javax.swing.Icon
import java.beans.PropertyEditor
import java.beans.PropertyEditorManager
...
Object iconValue = getApplication().getResourceResolver()
.resolveResource('menu.icon', ['large'])
PropertyEditor propertyEditor = PropertyEditorManager.findEditor(Icon)
propertyEditor.setAsText(String.valueOf(iconValue))
Icon icon = propertyEditor.getValue()
12.3.3. Reference Keys
There may be times where you would want to have a 2 keys reference the same value,
as if one key were an alias for the other. ResourceResolver
supports the notion of
referenced keys for this matter. In order to achieve this, the value of the alias
key must define the aliased key with a special format, for example
action.icon = /img/icons/action-{0}.png
hello.icon = @[action.icon]
Resolving those keys results in
assert getApplication()
.getResourceResolver()
.resolveResource('action.icon', ['copy']) == '/img/icons/action-copy.png'
assert getApplication()
.getResourceResolver()
.resolveResource('hello.icon', ['paste']) == '/img/icons/action-paste.png'
12.4. ResourceResolver Configuration
Resources may be configured in either properties files or Groovy scripts.
Groovy scripts have precedence over properties files should there be two files that
match the same basename
. The default configured basename
is "resources
", thus
the application will search for the following resources in the classpath.
-
resources.properties
-
resources.groovy
Of course Groovy scripts are only enabled if you add a dependency to the griffon-groovy
module to your project. The default basename
may be changed to some other value,
or additional basenames may be specified too; it’s just a matter of configuring a
Module override
@ServiceProviderFor(Module.class)
@Named("application")
@DependsOn("core")
public class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(ResourceResolver.class)
.withClassifier(named("applicationResourceResolver"))
.toProvider(new ResourceResolverProvider("custom_basename"))
.asSingleton();
}
}
Both properties files and Groovy scripts are subject locale aware loading mechanism
described next. For a Locate set to de_CH_Basel
the following resources will be
searched for and loaded
-
resources.properties
-
resources.groovy
-
resources_de.properties
-
resources_de.groovy
-
resources_de_CH.properties
-
resources_de_CH.groovy
-
resources_de_CH_Basel.properties
-
resources_de_CH_Basel.groovy
Properties files and Groovy scripts used for internationalization purposes are usually
placed under griffon-app/resources
The default resources.properties
file is placed in
this directory upon creating an application using the standard project templates.
12.5. The @ResourceResolverAware AST Transformation
Any component may gain the ability to resolve resources through a ResourceResolver
instance. You only need annotate the class with @griffon.transform.ResourceResolverAware
and it will automatically gain all methods exposed by ResourceResolver
.
This feature is just a shortcut to avoid reaching for the application instance from objects that do not hold a reference to it.
Here’s an example of a custom bean that’s able to resolve resources
@griffon.transform.ResourceResolverAware
class Bean {
String name
}
This class can be used in the following way
class SampleService {
@Inject Bean bean
String lookupValues(String arg) {
bean.name = arg
bean.resolveResource('some.resource.key', [bean.name])
}
}
The application’s ResourceResolver
will be injected to annotated beans if no name is
specified as an argument to ResourceResolverAware
. You may define multiple ResourceResolver
bindings as long as you qualify them with a distinct name, such as
@ServiceProviderFor(Module.class)
@Named("application")
public class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(ResourceResolver.class)
.withClassifier(AnnotationUtils.named("foo"))
.toProvider(new ResourceResolverProvider("foofile"))
.asSingleton();
bind(ResourceResolver.class)
.withClassifier(AnnotationUtils.named("bar"))
.toProvider(new ResourceResolverProvider("barfile"))
.asSingleton();
}
}
Then make use of any of these bindings like so
@griffon.transform.ResourceResolverAware('foo')
class Bean {
String name
}
12.6. Resource Injection
Resources may be automatically injected to any instance created via the application’s
Injector
. Injection points must be annotated with @griffon.core.resources.InjectedResource
which can be set on properties (Groovy), fields (Java and Groovy) and setter methods (Java and Groovy).
@InjectedResource is a perfect companion to models as the following example shows
sample.SampleModel.griffonLogo = /griffon-logo-48x48.png
logo = /griffon-logo-{0}x{0}.png
package sample
import griffon.core.resources.InjectedResource
import javax.swing.Icon
class SampleModel {
@InjectedResource Icon griffonLogo
@InjectedResource(key='logo', args=['16']) Icon smallGriffonLogo
@InjectedResource(key='logo', args=['64']) Icon largeGriffonLogo
}
@InjectedResource
assumes a naming convention in order to determine the resource key
to use. These are the rules applied by the default by ResourcesInjector
:
-
If a value is specified for the
key
argument then use it as is. -
otherwise construct a key based in the field name prefixed with the full qualified class name of the field’s owner.
You may also specify a default value if the resource definition is not found, however
be aware that this value must be set as a String thus guaranteeing a type conversion.
An optional format
value may be specified as a hint to the PropertyEditor used during
value conversion, for example
package sample
import griffon.core.resources.InjectedResource
class SampleModel {
@InjectedResource(defaultValue='10.04.2013 2:30 PM', format='dd.MM.yyyy h:mm a')
Date date
}
12.7. Property Editors
Resource injection makes use of the PropertyEditor
mechanism provided by the java.beans
package. The default ResourcesInjector
queries
PropertyEditorManager
whenever a resource value must be transformed to a target type.
PropertyEditorManager provides methods for registering custom PropertyEditors, it also
follows a class name convention to load PropertyEditors should a custom one is not
programmatically registered. Griffon applications will automatically load and register
PropertyEditors from the following classpath resource: /META-INF/services/java.beans.PropertyEditor
.
Each line follows the format
target.type = full.qualified.classname
The following table enumerates the default PropertyEditors loaded by Griffon at startup.
Plugins such as griffon-swing
and griffon-javafx
may register additional editors.
Type | Editor Class |
---|---|
java.lang.String |
griffon.core.editors.StringPropertyEditor |
java.io.File |
griffon.core.editors.FilePropertyEditor |
java.net.URL |
griffon.core.editors.URLPropertyEditor |
java.net.URI |
griffon.core.editors.URIPropertyEditor |
java.math.BigDecimal |
griffon.core.editors.BigDecimalPropertyEditor |
java.math.BigInteger |
griffon.core.editors.BigIntegerPropertyEditor |
java.lang.Boolean |
griffon.core.editors.BooleanPropertyEditor |
java.lang.Byte |
griffon.core.editors.BytePropertyEditor |
java.lang.Short |
griffon.core.editors.ShortPropertyEditor |
java.lang.Integer |
griffon.core.editors.IntegerPropertyEditor |
java.lang.Long |
griffon.core.editors.LongPropertyEditor |
java.lang.Float |
griffon.core.editors.FloatPropertyEditor |
java.lang.Double |
griffon.core.editors.DoublePropertyEditor |
java.util.Calendar |
griffon.core.editors.CalendarPropertyEditor |
java.util.Date |
griffon.core.editors.DatePropertyEditor |
15. Build Tools
15.1. GVM
From GVM’s website
GVM is a tool for managing parallel Versions of multiple Software Development Kits on most Unix based systems. It provides a convenient command line interface for installing, switching, removing and listing Candidates.
GVM can be used to install and keep up to date other build tools that make your life
easier when developing Griffon projects. These tools are lazybones
and gradle
.
Installing GVM itself is as easy as typing the following on a command prompt
$ curl -s get.gvmtool.net | bash
Next install the latest versions of lazybones
and gradle
by invoking
$ gvm install lazybones
$ gvm install gradle
GVM works on POSIX compliant environments, event on Windows if Cygwin is installed. We recommend you to install Babun shell as it enables much more features than plain Cygwin. There’s also a Power Shell alternative: posh-gvm.
15.2. Lazybones
Lazybones allows you to create a new project structure for any framework or library for which the tool has a template.
15.2.1. Configuration
All standard Griffon templates are published to https://bintray.com/griffon/griffon-lazybones-templates
You must configure this repository with Lazybones settings file. Edit $USER_HOME/.lazybones/config.groovy
and paste the following content
bintrayRepositories = [
"griffon/griffon-lazybones-templates",
"pledbrook/lazybones-templates"
]
Invoking the lazybones list
command should result in all currently available Griffon project
templates to be displayed in the output.
15.2.2. Templates
The following templates are available at the standard template repository
- griffon-swing-java
-
A template that initializes an application with Swing and Java.
- griffon-swing-groovy
-
A template that initializes an application with Swing and Groovy.
- griffon-javafx-ava
-
A template that initializes an application with JavaFX and Java.
- griffon-javafx-groovy
-
A template that initializes an application with JavaFX and Groovy.
- griffon-pivot-java
-
A template that initializes an application with Pivot and Java.
- griffon-pivot-groovy
-
A template that initializes an application with Pivot and Groovy.
- griffon-lanterna-java
-
A template that initializes an application with Lanterna and Java.
- griffon-lanterna-groovy
-
A template that initializes tan application with Lanterna and Groovy.
- griffon-plugin
-
A template that initializes a Griffon plugin project.
All application project templates include a subtemplate named artifact that provides the following artifact templates
- model
-
A standard Model artifact.
- view
-
A toolkit specific View artifact.
- controller
-
A standard Controller artifact.
- service
-
A standard Service artifact.
- test
-
A standard unit Test artifact.
- mvcgroup
-
Initializes Model, View, Controller, Test and IntegrationTest artifacts for a single MVC group.
The swing
and pivot
artifact templates provided additional artifact templates
- integrationTest
-
A standard integration Test artifact.
You can invoke any of these templates in the following ways
$ lazybones generate artifact::controller
This command creates a new Controller artifact. You’ll be asked for a package and a class name.
$ lazybones generate artifact::mvcgroup::com.acme::group
This command creates an MVC Group whose package is com.acme and class name is group. There will be 5 new artifacts in total.
15.3. Gradle
Gradle is the preferred build tool for a Griffon project. The Lazybones
templates create a default build.gradle
file that contains the minimum configuration
to build, test and package a Griffon application or plugin.
15.3.1. The "griffon" Plugin
The Griffon plugin adds default dependencies and conventional configuration to a Griffon project. This configuration follows the standard Griffon project layout.
Usage
To use the Griffon plugin, include in your build script:
buildscript {
repositories {
jcenter()
}
dependencies {
classpath 'org.codehaus.griffon:gradle-griffon-plugin:1.0.0.BETA2'
}
}
apply plugin: 'org.codehaus.griffon.griffon'
This plugin performs the following configurations when applied to a project
-
Register jcenter() as default repository.
-
Apply the following plugins:
idea
,java
,application
. -
Create additional compile-time only configurations: compileOnly, testCompileOnly.
-
Resolve plugin dependencies using the griffon configuration.
-
Adjusts javadoc/groovydoc/idea/eclipse classpaths given the new configurations.
-
Configures standard source directories with
main
andtest
source sets. -
Adjusts how
main
andtest
resource processing is performed.
The following dependencies are added by default too
-
on
compile
-
org.codehaus.griffon:griffon-core:2.0.0.BETA3
-
-
on
compileOnly
-
org.codehaus.griffon:griffon-core-compile:2.0.0.BETA3
-
-
on
testCompile
-
org.codehaus.griffon:griffon-core-test:2.0.0.BETA3
-
-
on
testCompileOnly
-
org.codehaus.griffon:griffon-core-compile:2.0.0.BETA3
-
If the toolkit conventional property is defined (plugins may opt to skip it) then the following dependencies are added
-
on
compile
-
org.codehaus.griffon:griffon-<toolkit>:2.0.0.BETA3
-
If the groovy
plugin is applied then the following dependencies are also added
-
on
compile
-
org.codehaus.griffon:griffon-groovy:2.0.0.BETA3
-
-
on
compile
-
org.codehaus.griffon:griffon-<toolkit>-groovy:2.0.0.BETA3
-
-
on
compileOnly
-
org.codehaus.griffon:griffon-groovy-compile:2.0.0.BETA3
-
-
on
testCompileOnly
-
org.codehaus.griffon:griffon-groovy-compile:2.0.0.BETA3
-
The griffon
configuration can be used to resolve dependencies using BOM files.
Assume for a moment that the griffon-scaffolding-plugin
is comprised of the following
modules
-
griffon-scaffolding - the core of the plugin, UI toolkit agnostic.
-
griffon-scaffolding-swing - Swing specific additions.
-
griffon-scaffolding-javafx-groovy - Groovy enhancements via
BuilderCustomizer
. -
griffon-scaffolding-javafx - JavaFX specific additions.
-
griffon-scaffolding-javafx-groovy - Groovy enhancements via
BuilderCustomizer
. -
griffon-scaffolding-groovy-compile - AST transformations.
As you can appreciate those are quite the set. You can manually define any of these dependencies on the build file, but given the many combinations it may be a bit hard to determine which dependencies should be added and which shouldn’t. The griffon configuration can make this decision for you; you just have to use it in the following way
dependencies {
griffon 'org.codehaus.griffon.plugins:griffon-scaffolding-plugin:0.0.0-SNAPSHOT'
}
This will add all required dependencies to your build by taking into account the project’s
choice of UI toolkit and whether the groovy
plugin has been applied or not. This
behavior can be configured and/or disabled by using the conventional properties
describe in the next section.
Convention properties
The Griffon plugin adds some properties to the project, which you can use to configure its behaviour.
Property name | Type | Default value | Description |
---|---|---|---|
disableDependencyResolution |
boolean |
false |
Disable automatic inclusion of dependencies defined with the griffon configuration. |
includeGroovyDependencies |
boolean |
- |
Force inclusion of Groovy dependencies defined with the griffon configuration. |
toolkit |
String |
_ |
The UI toolkit to use. May be left unset. Valid values are |
version |
String |
2.0.0.BETA3 |
The Griffon version to use for Griffon core dependencies. |
The includeGroovyDependencies property has 3 states: unset
, false
and true
.
Groovy dependencies will be added automatically to the project only if the value
of includeGroovyDependencies is unset
(default) and the groovy
plugin has been
applied to the project or if the value of includeGroovyDependencies is set to true
.
When the value of includeGroovyDependencies is set to false
then Groovy dependencies
will not be added, even if the groovy
plugin has been applied. This is useful for
Java projects that use Spock for testing, as you need the groovy
plugin in
order to compile Spock specifications but you wouldn’t want Groovy dependencies to
be pulled in for compilation.
15.3.2. The "griffon-build" Plugin
The Griffon Build plugin enables useful tasks required for plugin authors, such as the aggregation of Cobertura data files and reports; generation of a plugin BOM file, and more.
Usage
To use the Griffon Build plugin, include in your build script:
buildscript {
repositories {
jcenter()
}
dependencies {
classpath 'org.codehaus.griffon:gradle-griffon-build-plugin:1.0.0.BETA2'
}
}
apply plugin: 'org.codehaus.griffon.griffon-build'
Tasks
The Griffon Build plugin adds a number of tasks to your project, as shown below.
Task name | Depends on | Type | Description |
---|---|---|---|
aggregateCoberturaMerge |
- |
|
Aggregates all cobertura data files found in the project. |
aggregateCoberturaReport |
- |
|
Generates a project-wide Cobertura report. |
GenerateBomTask |
- |
|
Generates a BOM file that includes all subprojects. |
Convention Properties
The Griffon Build plugin adds a number of convention properties to the project, shown below.
Property name | Type | Default value | Description |
---|---|---|---|
coverageOutputDatafile |
File |
buildDir/cobertura/cobertura.ser |
Path to the data file to produce during instrumentation. |
coverageReportDir |
File |
reportsDir/cobertura |
Path to report directory for coverage report. |
16. Contributing
Griffon is an open source project with an active community and we rely heavily on that community to help make Griffon better. As such, there are various ways in which people can contribute to Griffon. One of these is by writing plugins and making them publicly available. In this chapter, we’ll look at some of the other options.
16.1. Issues
Griffon uses JIRA to track issues in the ore framework, its documentation, its website, and many of the public plugins. If you’ve found a bug or wish to see a particular feature added, this is the place to start. You’ll need to create a (free) JIRA account in order to either submit an issue or comment on an existing one.
When submitting issues, please provide as much information as possible and in the case of bugs, make sure you explain which versions of Griffon and various plugins you are using. Also, an issue is much more likely to be dealt with if you attach a reproducible sample application.
16.1.1. Reviewing issues
There are quite a few old issues in JIRA, some of which may no longer be valid. The core team can’t track down these alone, so a very simple contribution that you can make is to verify one or two issues occasionally.
Which issues need verification? A shared JIRA filter will display all issues that haven’t been resolved. Just pick one or two of them and check whether they are still relevant.
Once you’ve verified an issue, simply edit it by adding a "Last Reviewed" comment. If you think the issue can be closed, then also add a "Flagged" comment and a short explanation why.
16.2. Build
If you’re interested in contributing fixes and features to the core framework, you will have to learn how to get hold of the project’s source, build it and test it with your own applications. Before you start, make sure you have:
-
A JDK (1.7 or above)
-
A git client
Once you have all the pre-requisite packages installed, the next step is to download
the Griffon source code, which is hosted at GitHub in several
repositories owned by the griffon
GitHub user. This
is a simple case of cloning the repository you’re interested in. For example, to
get the core framework run:
$ git clone https://github.com/griffon/griffon.git
This will create a griffon
directory in your current working directory containing
all the project source files. The next step is to get a Griffon installation from the source.
16.2.1. Running the test suite
All you have to do to run the full suite of tests is:
$ ./gradlew test
These will take a while, so consider running individual tests using the command line. For example, to run the test case @MappingDslTests@ simply execute the following command:
$ ./gradlew -Dtest.single=EnvironmentTests :griffon-core:test
Note that you need to specify the sub-project that the test case resides in, because the top-level "test" target won’t work….
16.3. Patches
If you want to submit patches to the project, you simply need to fork the repository on GitHub rather than clone it directly. Then you will commit your changes to your fork and send a pull request for a core team member to review.
16.3.1. Forking and Pull Requests
One of the benefits of GitHub is the way that you can easily contribute to a project by forking the repository and sending pull requests with your changes.
What follows are some guidelines to help ensure that your pull requests are speedily dealt with and provide the information we need. They will also make your life easier!
Create a local branch for your changes
Your life will be greatly simplified if you create a local branch to make your changes on. For example, as soon as you fork a repository and clone the fork locally, execute
$ git checkout -b mine
This will create a new local branch called mine
based off the master
branch.
Of course, you can name the branch whatever you like - you don’t have to use mine
.
Create JIRAs for non-trivial changes
For any non-trivial changes, raise a JIRA issue if one doesn’t already exist. That helps us keep track of what changes go into each new version of Griffon.
Include JIRA issue ID in commit messages
This may not seem particularly important, but having a JIRA issue ID in a commit message means that we can find out at a later date why a change was made. Include the ID in any and all commits that relate to that issue. If a commit isn’t related to an issue, then there’s no need to include an issue ID.
Make sure your fork is up to date
Since the core developers must merge your commits into the main repository, it makes life much easier if your fork on GitHub is up to date before you send a pull request.
Let’s say you have the main repository set up as a remote called upstream
and you
want to submit a pull request. Also, all your changes are currently on the local mine
branch but not on master
. The first step involves pulling any changes from the main
repository that have been added since you last fetched and merged:
$ git checkout master
$ git pull upstream
This should complete without any problems or conflicts. Next, rebase your local branch against the now up-to-date master:
$ git checkout mine
$ git rebase master
What this does is rearrange the commits such that all of your changes come after the most recent one in master. Think adding some cards to the top of a deck rather than shuffling them into the pack.
You’ll now be able to do a clean merge from your local branch to master:
$ git checkout master
$ git merge mine
Finally, you must push your changes to your remote repository on GitHub, otherwise the core developers won’t be able to pick them up:
$ git push
You’re now ready to send the pull request from the GitHub user interface.
Say what your pull request is for
A pull request can contain any number of commits and it may be related to any number of issues. In the pull request message, please specify the IDs of all issues that the request relates to. Also give a brief description of the work you have done, such as: "I refactored the resources injector and added support for custom number editors (GRIFFON-xxxx)". :leveloffset: 1
Appendix A: Migrating from Griffon 1.x
Griffon 2.x has tried to be as close as possible to Griffon 1.x in terms of concepts and APIs, however some changes were introduced that require your attention when migrating an application from Griffon 1.x
1.1. Build Configuration
There is no longer a specific Griffon buildtime tool nor configuration settings. You must pick a build tool (we recommend Gradle) and use that tool’s configuration to match your needs. The simplest way to get started is to select an appropriate Lazybones template to create an empty project, then copy the files you need.
Build time plugins and scripts are now within the realm of a particular build tool.
1.1.1. Dependencies
Dependencies used to be configured inside griffon-app/conf/BuildConfig.groovy
. Now
that the file is gone you must configured dependencies using the native support of
the build tool you choose. Of particular note is that all griffon dependencies are
now standard JAR archives available from Maven compatible repositories, so they should
work no matter which dependency resolution you pick.
1.2. Runtime Configuration
1.2.1. Application and Config Scripts
The files Application.groovy
and Config.groovy
have been merged into a single
file : Config.groovy
. The log4j
DSL is no longer used, so please move your
logging settings to src/resources/log4j.properties
; you can also use the XML variant
if you want. Griffon 2.x does not forces Log4j on you either, you’re free to pick
a suitable Slf4j binding of your choice.
1.2.2. Builder Script
The Builder.groovy
is no longer required. Its functions are now handled by
BuilderCustomizer
classes bound in a Module
. You must add
griffon-groovy-2.0.0.BETA3.jar
as a compile dependency in order to use
BuilderCustomizers
. Here’s an example for adding a Miglayout
customization
package com.acme;
import griffon.inject.DependsOn;
import groovy.swing.factory.LayoutFactory;
import net.miginfocom.swing.MigLayout;
import groovy.util.Factory;
import org.codehaus.griffon.runtime.core.view.AbstractBuilderCustomizer;
import javax.inject.Named;
import java.util.LinkedHashMap;
import java.util.Map;
@Named("miglayout")
@DependsOn({"swing"})
@SuppressWarnings("rawtypes")
public class MiglayoutBuilderCustomizer extends AbstractBuilderCustomizer {
@SuppressWarnings("unchecked")
public MiglayoutBuilderCustomizer() {
Map<String, Factory> factories = new LinkedHashMap<>();
factories.put("miglayout", new LayoutFactory(MigLayout.class));
setFactories(factories);
}
}
package com.acme;
import griffon.core.injection.Module;
import griffon.inject.DependsOn;
import griffon.util.BuilderCustomizer;
import org.codehaus.griffon.runtime.core.injection.AbstractModule;
import org.kordamp.jipsy.ServiceProviderFor;
import javax.inject.Named;
@ServiceProviderFor(Module.class)
@DependsOn("swing-groovy")
@Named("miglayout-groovy")
public class MiglayoutModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(BuilderCustomizer.class)
.to(MiglayoutBuilderCustomizer.class)
.asSingleton();
}
}
1.2.3. Events Script
The Events
script usually placed in griffon-app/conf
should be moved to the main
source directory (src/main/java
or src/main/groovy
depending on your preferences).
The following snippet shows an skeleton implementation
package com.acme
import griffon.core.event.EventHandler
class ApplicationEventHandler implements EventHandler {
// event handlers as public methods
}
You must add event handlers as public methods following the conventions explained
in the consuming events section. Don’t forget to register this
class using a module. The default ApplicationModule
class provided by all basic
project templates is a good start.
package com.acme
import griffon.core.event.EventHandler
import griffon.core.injection.Module
import org.codehaus.griffon.runtime.core.injection.AbstractModule
import org.kordamp.jipsy.ServiceProviderFor
@ServiceProviderFor(Module)
class ApplicationModule extends AbstractModule {
@Override
protected void doConfigure() {
bind(EventHandler)
.to(ApplicationEventHandler)
.asSingleton()
}
}
1.3. Artifacts
All artifacts must be annotated with @ArtifactProviderFor
without exception.
Failure to follow this rule will make Griffon miss the artifact during bootstrap.
The value for this annotation must be the basic interface that defines the artifact’s
type, for example
package sample
import griffon.core.artifact.GriffonModel
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonModel)
class SampleModel {
...
}
1.3.1. Controllers
Closure properties as actions are no longer supported. All actions must be defined as public methods.
1.3.2. Views
View scripts have been upgraded to classes. You can use the following skeleton View class as an starting point
package sample
import griffon.core.artifact.GriffonView
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonView)
class SampleView {
def builder
def model
void initUI() {
builder.with {
(1)
}
}
}
1 | UI components |
Next, place the contents of your old View script inside 1.
1.4. Lifecycle Scripts
These scripts must be migrated to full classes too. Here’s the basic skeleton code for any lifecycle handler
import griffon.core.GriffonApplication
import org.codehaus.griffon.runtime.core.AbstractLifecycleHandler
import javax.annotation.Nonnull
import javax.inject.Inject
class Initialize extends AbstractLifecycleHandler {
@Inject
Initialize(@Nonnull GriffonApplication application) {
super(application)
}
@Override
void execute() {
// do the work
}
}
1.5. Tests
Griffon 2.x no longer segregates tests between unit
and functional
. You must use
your build tool’s native support for both types (this is quite simple with Gradle).
Move all unit tests under src/test/java
or src/test/groovy
depending on your
choice of main language. The base GriffonUnitTestCase
class no longer exist. Use
any testing framework you’re comfortable with to write unit tests (Junit4, Spock, etc).
Use the following template if you need to write artifact tests
package sample
import griffon.core.test.GriffonUnitRule
import griffon.core.test.TestFor
import org.junit.Rule
import org.junit.Test
import static com.jayway.awaitility.Awaitility.await
import static java.util.concurrent.TimeUnit.SECONDS
@TestFor(SampleController)
class SampleControllerTest {
private SampleController controller
@Rule
public final GriffonUnitRule griffon = new GriffonUnitRule()
@Test
void testControllerAction() {
// given:
// setup collaborators
// when:
// stimulus
controller.invokeAction('nameOfTheAction')
// then:
// use Awaitility to successfully wait for async processing to finish
await().atMost(2, SECONDS)
assert someCondition
}
}
Appendix B: Module Bindings
The following sections display all bindings per module. Use this information to successfully override a binding on your own modules or to troubleshoot a module binding if the wrong type has been applied by the Griffon runtime.
1.1. Core
Module name: core
bind(ApplicationClassLoader.class)
.to(DefaultApplicationClassLoader.class)
.asSingleton();
bind(ApplicationConfigurer.class)
.to(DefaultApplicationConfigurer.class)
.asSingleton();
bind(ResourceHandler.class)
.to(DefaultResourceHandler.class)
.asSingleton();
bind(CompositeResourceBundleBuilder.class)
.to(DefaultCompositeResourceBundleBuilder.class)
.asSingleton();
bind(ResourceBundle.class)
.withClassifier(named("applicationResourceBundle"))
.toProvider(new ResourceBundleProvider("Config"))
.asSingleton();
bind(Configuration.class)
.to(DefaultConfiguration.class)
.asSingleton();
bind(ExecutorServiceManager.class)
.to(DefaultExecutorServiceManager.class)
.asSingleton();
bind(EventRouter.class)
.withClassifier(named("applicationEventRouter"))
.to(DefaultEventRouter.class)
.asSingleton();
bind(EventRouter.class)
.to(DefaultEventRouter.class);
bind(ResourceResolver.class)
.withClassifier(named("applicationResourceResolver"))
.toProvider(new ResourceResolverProvider("resources"))
.asSingleton();
bind(MessageSource.class)
.withClassifier(named("applicationMessageSource"))
.toProvider(new MessageSourceProvider("messages"))
.asSingleton();
bind(ResourceInjector.class)
.withClassifier(named("applicationResourceInjector"))
.to(DefaultApplicationResourceInjector.class)
.asSingleton();
bind(UIThreadManager.class)
.to(DefaultUIThreadManager.class)
.asSingleton();
bind(MVCGroupManager.class)
.to(DefaultMVCGroupManager.class)
.asSingleton();
for (Lifecycle lifecycle : Lifecycle.values()) {
bind(LifecycleHandler.class)
.withClassifier(named(lifecycle.getName()))
.toProvider(new LifecycleHandlerProvider(lifecycle.getName()))
.asSingleton();
}
bind(WindowManager.class)
.to(NoopWindowManager.class)
.asSingleton();
bind(ActionManager.class)
.to(DefaultActionManager.class)
.asSingleton();
bind(ArtifactManager.class)
.to(DefaultArtifactManager.class)
.asSingleton();
bind(ArtifactHandler.class)
.to(ModelArtifactHandler.class)
.asSingleton();
bind(ArtifactHandler.class)
.to(ViewArtifactHandler.class)
.asSingleton();
bind(ArtifactHandler.class)
.to(ControllerArtifactHandler.class)
.asSingleton();
bind(ArtifactHandler.class)
.to(ServiceArtifactHandler.class)
.asSingleton();
bind(PlatformHandler.class)
.toProvider(PlatformHandlerProvider.class)
.asSingleton();
bind(AddonManager.class)
.to(DefaultAddonManager.class)
.asSingleton();
bind(EventHandler.class)
.to(DefaultEventHandler.class)
.asSingleton();
bind(GriffonExceptionHandler.class)
.asSingleton();
1.2. Groovy
Module name: groovy
bind(ConfigReader.class)
.toProvider(ConfigReader.Provider.class)
.asSingleton();
bind(CompositeResourceBundleBuilder.class)
.to(GroovyAwareCompositeResourceBundleBuilder.class)
.asSingleton();
bind(GriffonAddon.class)
.to(GroovyAddon.class)
.asSingleton();
bind(MVCGroupManager.class)
.to(GroovyAwareMVCGroupManager.class)
.asSingleton();
bind(BuilderCustomizer.class)
.to(CoreBuilderCustomizer.class)
.asSingleton();
1.3. Swing
Module name: swing
bind(SwingWindowDisplayHandler.class)
.withClassifier(named("defaultWindowDisplayHandler"))
.to(DefaultSwingWindowDisplayHandler.class)
.asSingleton();
bind(SwingWindowDisplayHandler.class)
.withClassifier(named("windowDisplayHandler"))
.to(ConfigurableSwingWindowDisplayHandler.class)
.asSingleton();
bind(WindowManager.class)
.to(DefaultSwingWindowManager.class)
.asSingleton();
bind(UIThreadManager.class)
.to(SwingUIThreadManager.class)
.asSingleton();
bind(ActionManager.class)
.to(SwingActionManager.class)
.asSingleton();
bind(GriffonAddon.class)
.to(SwingAddon.class)
.asSingleton();
1.4. Swing Builder
Module name: swing-groovy
Depends on: swing
bind(BuilderCustomizer.class)
.to(SwingBuilderCustomizer.class)
.asSingleton();
bind(SwingWindowDisplayHandler.class)
.withClassifier(named("windowDisplayHandler"))
.to(GroovyAwareConfigurableSwingWindowDisplayHandler.class)
.asSingleton();
1.5. JavaFX
Module name: javafx
bind(JavaFXWindowDisplayHandler.class)
.withClassifier(named("defaultWindowDisplayHandler"))
.to(DefaultJavaFXWindowDisplayHandler.class)
.asSingleton();
bind(JavaFXWindowDisplayHandler.class)
.withClassifier(named("windowDisplayHandler"))
.to(ConfigurableJavaFXWindowDisplayHandler.class)
.asSingleton();
bind(WindowManager.class)
.to(DefaultJavaFXWindowManager.class)
.asSingleton();
bind(UIThreadManager.class)
.to(JavaFXUIThreadManager.class)
.asSingleton();
bind(ActionManager.class)
.to(JavaFXActionManager.class)
.asSingleton();
1.6. JavaFX Builder
Module name: javafx-groovy
Depends on: javafx
bind(BuilderCustomizer.class)
.to(JavafxBuilderCustomizer.class)
.asSingleton();
bind(JavaFXWindowDisplayHandler.class)
.withClassifier(named("windowDisplayHandler"))
.to(GroovyAwareConfigurableJavaFXWindowDisplayHandler.class)
.asSingleton();
1.7. Lanterna
Module name: lanterna
bind(GUIScreen.class)
.toProvider(GUIScreenProvider.class)
.asSingleton();
bind(LanternaWindowDisplayHandler.class)
.withClassifier(named("defaultWindowDisplayHandler"))
.to(DefaultLanternaWindowDisplayHandler.class)
.asSingleton();
bind(LanternaWindowDisplayHandler.class)
.withClassifier(named("windowDisplayHandler"))
.to(ConfigurableLanternaWindowDisplayHandler.class)
.asSingleton();
bind(WindowManager.class)
.to(DefaultLanternaWindowManager.class)
.asSingleton();
bind(UIThreadManager.class)
.to(LanternaUIThreadManager.class)
.asSingleton();
bind(ActionManager.class)
.to(LanternaActionManager.class)
.asSingleton();
1.8. Lanterna Builder
Module name: lanterna-groovy
Depends on: lanterna
bind(BuilderCustomizer.class)
.to(LanternaBuilderCustomizer.class)
.asSingleton();
bind(LanternaWindowDisplayHandler.class)
.withClassifier(named("windowDisplayHandler"))
.to(GroovyAwareConfigurableLanternaWindowDisplayHandler.class)
.asSingleton();
1.9. Pivot
Module name: pivot
bind(PivotWindowDisplayHandler.class)
.withClassifier(named("defaultWindowDisplayHandler"))
.to(DefaultPivotWindowDisplayHandler.class)
.asSingleton();
bind(PivotWindowDisplayHandler.class)
.withClassifier(named("windowDisplayHandler"))
.to(ConfigurablePivotWindowDisplayHandler.class)
.asSingleton();
bind(WindowManager.class)
.to(DefaultPivotWindowManager.class)
.asSingleton();
bind(UIThreadManager.class)
.to(PivotUIThreadManager.class)
.asSingleton();
bind(ActionManager.class)
.to(PivotActionManager.class)
.asSingleton();
1.10. Pivot Builder
Module name: pivot-groovy
Depends on: pivot
bind(BuilderCustomizer.class)
.to(PivotBuilderCustomizer.class)
.asSingleton();
bind(PivotWindowDisplayHandler.class)
.withClassifier(named("windowDisplayHandler"))
.to(GroovyAwareConfigurablePivotWindowDisplayHandler.class)
.asSingleton();
Appendix C: AST Transformations
The following list summarizes all AST transformations available to Groovy based
projects when the griffon-groovy-compile-2.0.0.BETA3.jar
dependency is
added to a project
Appendix D: Sample Applications
This appendix showcases the same application implemented with different languages
and different UI toolkits. The application presents a very simple form where a user
is asked for his or her name. Once a button is clicked a reply will appear within
the same window. In order to achieve this Models hold 2 observable properties: the
first to keep track of the input
, the second to do the same for the output
.
Views are only concerned with values coming from the model and as such never
interact directly with Controllers. Controllers in turn only interact with Models
and a Service used to transform the input value into the output value. The single
controller action observes the rules for invoking computations outside of the UI
thread and updating UI components inside the UI thread.
These are some screenshots of each one of the applications we’ll cover next.
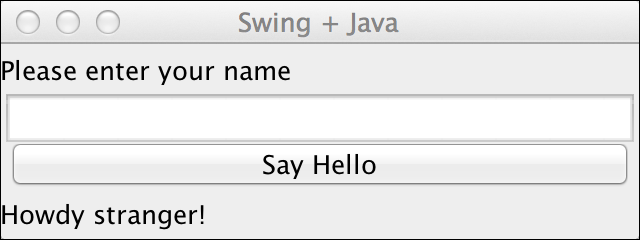
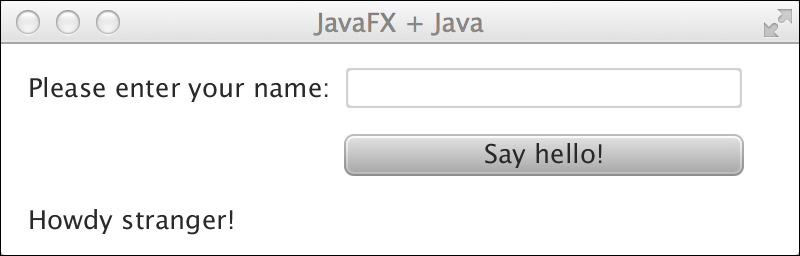
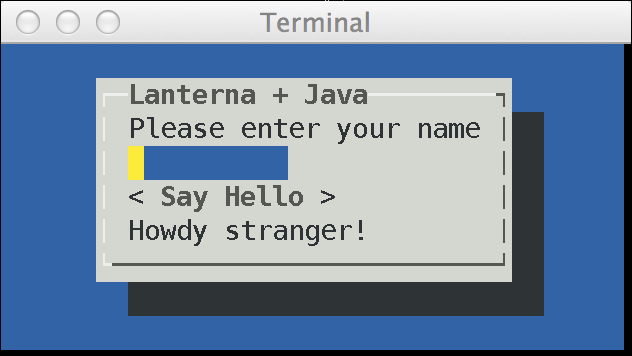
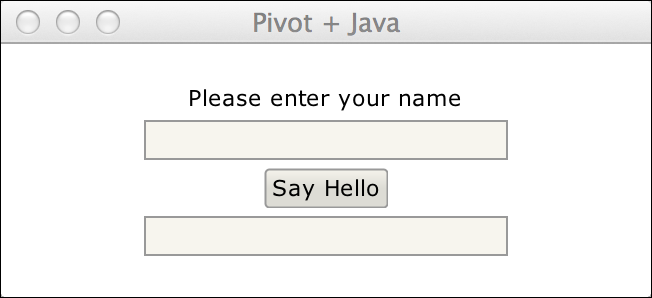
The goal of these applications is to showcase the similarities and differences of each one of them given their implementation language and UI toolkit.
1.1. Swing
Let’s begin with Swing, as it’s probably the most well known Java UI toolkit. First we’ll show the Java version of an artifact, then we’ll show it’s Groovy counterpart.
1.1.1. Model
Instances of GriffonModel
implement the Observable
interface which
means they know how to handle observable properties out of the box. We only need to be
concerned in triggering a java.beans.PropertyChangeEvent
when a property changes value.
package sample.swing.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonModel;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonModel;
import javax.annotation.Nonnull;
import javax.inject.Inject;
@ArtifactProviderFor(GriffonModel.class)
public class SampleModel extends AbstractGriffonModel {
private String input; (1)
private String output; (1)
@Inject
public SampleModel(@Nonnull GriffonApplication application) {
super(application);
}
public String getInput() { (2)
return input;
}
public void setInput(String input) {
firePropertyChange("input", this.input, this.input = input); (3)
}
public String getOutput() { (2)
return output;
}
public void setOutput(String output) {
firePropertyChange("output", this.output, this.output = output); (3)
}
}
1 | Define a private field for the property |
2 | Property accessor |
3 | Property mutator must fire a PropertyChangeEvent |
The code is quite straight forward, there’s nothing much to see here other than making sure to follow the rules for creating observable properties. The Groovy version sports a short hand thanks to the usage of the @Observable AST transformation.
One key difference between the Java and the Groovy version is that the Groovy Model
does not extend a particular class. It also This is due to Griffon being aware of its own
conventions and applying the appropriate byte code manipulation (via AST transformations).
The compiled Model class does implement the GriffonModel
interface as required by
the framework. This type of byte code manipulation is expected to work for every Groovy
based artifact.
package sample.swing.groovy
import griffon.core.artifact.GriffonModel
import griffon.metadata.ArtifactProviderFor
import griffon.transform.Observable
@ArtifactProviderFor(GriffonModel)
class SampleModel {
@Observable String input (1)
@Observable String output (1)
}
1 | Observable property |
Properties become observable by simply annotating them with @Observable. The Groovy compiler will generate the required boilerplate code, which so happens to be functionally equivalent to what we showed in the Java version.
1.1.2. Controller
Controllers provide actions that are used to fill up the application’s interaction.
They usually manipulate values coming from Views via Model properties. Controllers may
rely on additional components, such as Services, to do they work. This is exactly our
case as there’s a SampleService
instance injected into our controllers.
package sample.swing.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonController;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonController;
import javax.annotation.Nonnull;
import javax.inject.Inject;
@ArtifactProviderFor(GriffonController.class)
public class SampleController extends AbstractGriffonController {
private SampleModel model; (1)
@Inject
private SampleService sampleService; (2)
@Inject
public SampleController(@Nonnull GriffonApplication application) {
super(application);
}
public void setModel(SampleModel model) {
this.model = model;
}
public void sayHello() { (3)
final String result = sampleService.sayHello(model.getInput());
runInsideUIAsync(new Runnable() { (4)
@Override
public void run() {
model.setOutput(result);
}
});
}
}
1 | MVC member injected by MVCGroupManager |
2 | Injected by JSR 330 |
3 | Automatically run off the UI thread |
4 | Get back inside the UI thread |
Of particular note is the fact that actions are always executed outside off the UI thread
unless otherwise configured with an @Threading
annotation. Once we have computed the right
output we must inform the View of the new value. This is done by updating the model
inside the UI thread 4.
package sample.swing.groovy
import griffon.core.artifact.GriffonController
import griffon.metadata.ArtifactProviderFor
import javax.inject.Inject
@ArtifactProviderFor(GriffonController)
class SampleController {
SampleModel model (1)
@Inject
private SampleService sampleService (2)
void sayHello() { (3)
String result = sampleService.sayHello(model.input)
model.output = result (4)
}
}
1 | MVC member injected by MVCGroupManager |
2 | Injected by JSR 330 |
3 | Automatically run off the UI thread |
4 | Get back inside the UI thread |
The Groovy version of the Controller is much terser of course however there’s a nice feature available to Groovy Swing: Model properties bound to UI components are always updated inside the UI thread.
1.1.3. Service
Services are tasked to work with raw data and I/O, they should never interact with
Views and Models directly, though you may have additional components injected to them.
The following service shows another facility provide by the
GriffonApplication
interface:
MessageSource
, capable of resolving i18n-able
resources.
package sample.swing.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonService;
import griffon.core.i18n.MessageSource;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonService;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import static griffon.util.GriffonNameUtils.isBlank;
import static java.util.Arrays.asList;
@ArtifactProviderFor(GriffonService.class)
public class SampleService extends AbstractGriffonService {
@Inject
public SampleService(@Nonnull GriffonApplication application) {
super(application);
}
public String sayHello(String input) {
MessageSource messageSource = getApplication().getMessageSource();
if (isBlank(input)) {
return messageSource.getMessage("greeting.default");
} else {
return messageSource.getMessage("greeting.parameterized", asList(input));
}
}
}
package sample.swing.groovy
import griffon.core.artifact.GriffonService
import griffon.core.i18n.MessageSource
import griffon.metadata.ArtifactProviderFor
import static griffon.util.GriffonNameUtils.isBlank
@ArtifactProviderFor(GriffonService)
class SampleService {
String sayHello(String input) {
MessageSource ms = application.messageSource
isBlank(input) ? ms.getMessage('greeting.default') : ms.getMessage('greeting.parameterized', [input])
}
}
1.1.4. View
We come to the final piece of the puzzle: the View. Components are arranged in a one column vertical grid
package sample.swing.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonView;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.swing.artifact.AbstractSwingGriffonView;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import javax.swing.*;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import java.awt.GridLayout;
import java.awt.Image;
import java.awt.Toolkit;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.Collections;
import static java.util.Arrays.asList;
import static javax.swing.WindowConstants.DO_NOTHING_ON_CLOSE;
@ArtifactProviderFor(GriffonView.class)
public class SampleView extends AbstractSwingGriffonView {
private SampleController controller; (1)
private SampleModel model; (1)
@Inject
public SampleView(@Nonnull GriffonApplication application) {
super(application);
}
public void setController(SampleController controller) {
this.controller = controller;
}
public void setModel(SampleModel model) {
this.model = model;
}
@Override
public void initUI() {
JFrame window = (JFrame) getApplication()
.createApplicationContainer(Collections.<String, Object>emptyMap());
window.setName("mainWindow");
window.setTitle(getApplication().getConfiguration().getAsString("application.title"));
window.setSize(320, 120);
window.setDefaultCloseOperation(DO_NOTHING_ON_CLOSE);
window.setIconImage(getImage("/griffon-icon-48x48.png"));
window.setIconImages(asList(
getImage("/griffon-icon-48x48.png"),
getImage("/griffon-icon-32x32.png"),
getImage("/griffon-icon-16x16.png")
));
getApplication().getWindowManager().attach("mainWindow", window); (2)
window.getContentPane().setLayout(new GridLayout(4, 1));
window.getContentPane().add(
new JLabel(getApplication().getMessageSource().getMessage("name.label"))
);
final JTextField nameField = new JTextField();
nameField.setName("inputField");
nameField.getDocument().addDocumentListener(new DocumentListener() { (3)
@Override
public void insertUpdate(DocumentEvent e) {
model.setInput(nameField.getText());
}
@Override
public void removeUpdate(DocumentEvent e) {
model.setInput(nameField.getText());
}
@Override
public void changedUpdate(DocumentEvent e) {
model.setInput(nameField.getText());
}
});
window.getContentPane().add(nameField);
Action action = toolkitActionFor(controller, "sayHello"); (4)
final JButton button = new JButton(action);
button.setName("sayHelloButton");
window.getContentPane().add(button);
final JLabel outputLabel = new JLabel();
outputLabel.setName("outputLabel");
model.addPropertyChangeListener("output", new PropertyChangeListener() { (3)
@Override
public void propertyChange(PropertyChangeEvent evt) {
outputLabel.setText(String.valueOf(evt.getNewValue()));
}
});
window.getContentPane().add(outputLabel);
}
private Image getImage(String path) {
return Toolkit.getDefaultToolkit().getImage(SampleView.class.getResource(path));
}
}
1 | MVC member injected by MVCGroupManager |
2 | Attach window to WindowManager |
3 | Apply component-to-model binding |
4 | Hook in controller action by name |
Here we can appreciate at 3 how Model properties are bound to View components, also how controller actions can be transformed into toolkit actions that may be applied to buttons 4 for example.
package sample.swing.groovy
import griffon.core.artifact.GriffonView
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonView)
class SampleView {
FactoryBuilderSupport builder (1)
SampleModel model (1)
void initUI() {
builder.with {
application(title: application.configuration['application.title'], (2)
id: 'mainWindow', size: [320, 160],
iconImage: imageIcon('/griffon-icon-48x48.png').image,
iconImages: [imageIcon('/griffon-icon-48x48.png').image,
imageIcon('/griffon-icon-32x32.png').image,
imageIcon('/griffon-icon-16x16.png').image]) {
gridLayout(rows: 4, cols: 1)
label(application.messageSource.getMessage('name.label'))
textField(id: 'inputField', text: bind(target: model, 'input')) (3)
button(sayHelloAction, id: 'sayHelloButton') (4)
label(id: 'outputLabel', text: bind { model.output }) (3)
}
}
}
}
1 | MVC member injected by MVCGroupManager |
2 | Create window and attach it to WindowManager |
3 | Apply component-to-model binding |
4 | Hook in controller action by name |
The Groovy version is again much terser thanks to the SwingBuilder DSL.
Notice how easy it’s to bind 3 model properties using the +bind+ node.
The controller action is also transformed into an UI toolkit specific action however
this time it’s easier to grab: by convention all controller actions are exposed as variables
to the corresponding builder
.
1.1.5. Resources
The last file we’ll touch is the one that holds de i18n-able content. Griffon supports several formats. Here we’re showing the standard one as found in many Java projects.
name.label = Please enter your name
greeting.default = Howdy stranger!
greeting.parameterized = Hello {0}
1.2. JavaFX
JavaFx is a next generation UI toolkit and will eventually replace Swing, so it’s a good idea to get started with it now. Among the several features found in JavaFX you’ll find
-
observable properties
-
component styling with CSS
-
FXML: a declarative format for defining UIs
-
and more!
1.2.1. Model
package sample.javafx.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonModel;
import griffon.metadata.ArtifactProviderFor;
import javafx.beans.property.SimpleStringProperty;
import javafx.beans.property.StringProperty;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonModel;
import javax.annotation.Nonnull;
import javax.inject.Inject;
@ArtifactProviderFor(GriffonModel.class)
public class SampleModel extends AbstractGriffonModel {
private StringProperty input; (1)
private StringProperty output; (1)
@Inject
public SampleModel(@Nonnull GriffonApplication application) {
super(application);
}
@Nonnull
public final StringProperty inputProperty() { (2)
if (input == null) {
input = new SimpleStringProperty(this, "input");
}
return input;
}
public void setInput(String input) { (3)
inputProperty().set(input);
}
public String getInput() { (3)
return input == null ? null : inputProperty().get();
}
@Nonnull
public final StringProperty outputProperty() { (2)
if (output == null) {
output = new SimpleStringProperty(this, "output");
}
return output;
}
public void setOutput(String output) { (3)
outputProperty().set(output);
}
public String getOutput() { (3)
return output == null ? null : outputProperty().get();
}
}
1 | Define a private field for the property |
2 | Property accessor |
3 | Pass-thru values to Property |
The Model makes use of JavaFX’s observable properties .These properties are roughly equivalent in behavior to the ones we saw back in the Swing example, however they provide much more behavior than that; values may be cached automatically for example, avoiding bindings to be updated needlessly.
package sample.javafx.groovy
import griffon.core.artifact.GriffonModel
import griffon.metadata.ArtifactProviderFor
import griffon.transform.FXObservable
@ArtifactProviderFor(GriffonModel)
class SampleModel {
@FXObservable String input (1)
@FXObservable String output (1)
}
1 | Observable property |
Similarly to @Observable
we find that Groovy based JavaFX models can use another AST
transformation named @FXObservable
. This transformation generates equivalent byte code
to the Java based Model we saw earlier.
1.2.2. Controller
Have a look at the controller for this application. See if you can spot the differences between its Swing counterpart.
package sample.javafx.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonController;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonController;
import javax.annotation.Nonnull;
import javax.inject.Inject;
@ArtifactProviderFor(GriffonController.class)
public class SampleController extends AbstractGriffonController {
private SampleModel model; (1)
@Inject
private SampleService sampleService; (2)
@Inject
public SampleController(@Nonnull GriffonApplication application) {
super(application);
}
public void setModel(SampleModel model) {
this.model = model;
}
public void sayHello() { (3)
final String result = sampleService.sayHello(model.getInput());
runInsideUIAsync(new Runnable() { (4)
@Override
public void run() {
model.setOutput(result);
}
});
}
}
1 | MVC member injected by MVCGroupManager |
2 | Injected by JSR 330 |
3 | Automatically run off the UI thread |
4 | Get back inside the UI thread |
Di you spot any differences? No? That’s because the two controllers are 100% identical. How can this be? This is the power of separating concerns between MVC members. Because the Controller only talks to the View via the Model, and the Model exposes an identical contract for its properties we didn’t have to change the Controller at all.
package sample.javafx.groovy
import griffon.core.artifact.GriffonController
import griffon.metadata.ArtifactProviderFor
import javax.inject.Inject
@ArtifactProviderFor(GriffonController)
class SampleController {
SampleModel model (1)
@Inject
private SampleService sampleService (2)
void sayHello() { (3)
String result = sampleService.sayHello(model.input)
runInsideUIAsync { (4)
model.output = result
}
}
}
1 | MVC member injected by MVCGroupManager |
2 | Injected by JSR 330 |
3 | Automatically run off the UI thread |
4 | Get back inside the UI thread |
Opposed to its Swing counterpart here we have to add an explicit threading block when
updating model properties. This is because the bind
node for JavaFX components is not
aware of the same rules than the bind
node for Swing components. Nevertheless the
code remains short and to the point.
1.2.3. Service
Given that the service operates with raw data and has no ties to the toolkit in use we’d expect no changes from the Swing example.
package sample.javafx.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonService;
import griffon.core.i18n.MessageSource;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonService;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import static griffon.util.GriffonNameUtils.isBlank;
import static java.util.Arrays.asList;
@ArtifactProviderFor(GriffonService.class)
public class SampleService extends AbstractGriffonService {
@Inject
public SampleService(@Nonnull GriffonApplication application) {
super(application);
}
public String sayHello(String input) {
MessageSource messageSource = getApplication().getMessageSource();
if (isBlank(input)) {
return messageSource.getMessage("greeting.default");
} else {
return messageSource.getMessage("greeting.parameterized", asList(input));
}
}
}
package sample.javafx.groovy
import griffon.core.artifact.GriffonService
import griffon.core.i18n.MessageSource
import griffon.metadata.ArtifactProviderFor
import static griffon.util.GriffonNameUtils.isBlank
@ArtifactProviderFor(GriffonService)
class SampleService {
String sayHello(String input) {
MessageSource ms = application.messageSource
isBlank(input) ? ms.getMessage('greeting.default') : ms.getMessage('greeting.parameterized', [input])
}
}
1.2.4. View
Views are the artifacts that are most impacted by the choice of UI toolkit. You may remember we mentioned FXML as one of the strong features delivered by JavaFX and so we chose to implement the Java based View by reading an fxml file by convention.
package sample.javafx.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonView;
import griffon.metadata.ArtifactProviderFor;
import javafx.fxml.FXML;
import javafx.scene.Group;
import javafx.scene.Node;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import org.codehaus.griffon.runtime.javafx.artifact.AbstractJavaFXGriffonView;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import java.util.Collections;
@ArtifactProviderFor(GriffonView.class)
public class SampleView extends AbstractJavaFXGriffonView {
private SampleController controller; (1)
private SampleModel model; (1)
@FXML
private TextField input; (2)
@FXML
private Label output; (2)
@Inject
public SampleView(@Nonnull GriffonApplication application) {
super(application);
}
public void setController(SampleController controller) {
this.controller = controller;
}
public void setModel(SampleModel model) {
this.model = model;
}
@Override
public void initUI() {
Stage stage = (Stage) getApplication()
.createApplicationContainer(Collections.<String,Object>emptyMap());
stage.setTitle(getApplication().getConfiguration().getAsString("application.title"));
stage.setWidth(400);
stage.setHeight(120);
stage.setScene(init());
getApplication().getWindowManager().attach("mainWindow", stage); (3)
}
// build the UI
private Scene init() {
Scene scene = new Scene(new Group());
scene.setFill(Color.WHITE);
Node node = loadFromFXML();
model.inputProperty().bindBidirectional(input.textProperty());
model.outputProperty().bindBidirectional(output.textProperty());
((Group) scene.getRoot()).getChildren().addAll(node);
connectActions(node, controller); (4)
return scene;
}
}
1 | MVC member injected by MVCGroupManager |
2 | Create window and attach it to WindowManager |
3 | Injected by FXMLLoader |
4 | Hook actions by convention |
FXMLLoader
can inject components to an instance as long as that instance exposes
fields annotated with @FXML
; fields names must match component ids 2
as defined in the fxml file, which is shown next:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.control.TextField?>
<?import javafx.scene.layout.AnchorPane?>
<AnchorPane id="AnchorPane" maxHeight="-Infinity" maxWidth="-Infinity"
minHeight="-Infinity" minWidth="-Infinity"
prefHeight="80.0" prefWidth="384.0"
xmlns:fx="http://javafx.com/fxml"
fx:controller="sample.javafx.java.SampleController">
<children>
<Label layoutX="14.0" layoutY="14.0" text="Please enter your name:"/>
<TextField fx:id="input" layoutX="172.0" layoutY="11.0"
prefWidth="200.0"/>
<Button layoutX="172.0" layoutY="45.0"
mnemonicParsing="false"
prefWidth="200.0"
text="Say hello!"
fx:id="sayHelloActionTarget" /> (1)
<Label layoutX="14.0" layoutY="80.0" prefWidth="360.0" fx:id="output"/>
</children>
</AnchorPane>
1 | Naming convention for automatic action binding |
Please pay special attention to the fx:id
given to the button. Griffon applies a naming
convention to match controller actions to JavaFX components that can handle said actions.
Let’s review what we have here:
-
SampleController
exposes an action namedsayHello
-
the button has an
fx:id
value ofsayHelloActionTarget
Given this we infer that the fx:id
value must be of the form <actionName>ActionTarget
.
The naming convention is one of two steps, you must also connect the controller using
a helper method 4 as shown in the View.
package sample.javafx.groovy
import griffon.core.artifact.GriffonView
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonView)
class SampleView {
FactoryBuilderSupport builder (1)
SampleModel model (1)
void initUI() {
builder.application(title: application.configuration['application.title'],
sizeToScene: true, centerOnScreen: true) { (2)
scene(fill: WHITE, width: 400, height: 120) {
anchorPane {
label(leftAnchor: 14, topAnchor: 11,
text: application.messageSource.getMessage('name.label'))
textField(leftAnchor: 172, topAnchor: 11, prefWidth: 200,
text: bind(model.inputProperty())) (3)
button(leftAnchor: 172, topAnchor: 45, prefWidth: 200,
sayHelloAction) (4)
label(leftAnchor: 14, topAnchor: 80, prefWidth: 200,
text: bind(model.outputProperty())) (3)
}
}
}
}
}
1 | MVC member injected by MVCGroupManager |
2 | Create window and attach it to WindowManager |
3 | Apply component-to-model binding |
4 | Hook actions by convention |
The Groovy version of the View uses the GroovyFX DSL instead of FXML. You’ll find that this DSL is very similar to SwingBuilder.
1.3. Lanterna
Lanterna is a Java library allowing you to write easy semi-graphical user interfaces in a text-only environment, very similar to the C library curses but with more functionality. Lanterna supports xterm compatible terminals and terminal emulators such as konsole, gnome-terminal, putty, xterm and many more. One of the main benefits of lanterna is that it’s not dependent on any native library but runs 100% in pure Java.
1.3.1. Model
Even though Lanterna UI components do not expose observable properties in any way it’s a good thing to use observable properties in the Model, and so the following Model is identical to the Swing version.
package sample.lanterna.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonModel;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonModel;
import javax.annotation.Nonnull;
import javax.inject.Inject;
@ArtifactProviderFor(GriffonModel.class)
public class SampleModel extends AbstractGriffonModel {
private String input; (1)
private String output; (1)
@Inject
public SampleModel(@Nonnull GriffonApplication application) {
super(application);
}
public String getInput() { (2)
return input;
}
public void setInput(String input) {
firePropertyChange("input", this.input, this.input = input); (3)
}
public String getOutput() { (2)
return output;
}
public void setOutput(String output) {
firePropertyChange("output", this.output, this.output = output); (3)
}
}
1 | Define a private field for the property |
2 | Property accessor |
3 | Property mutator must fire a PropertyChangeEvent |
For reasons we’ll see in the Groovy View and Controller we decided to skip a Model for the Groovy version. This also demonstrates that even though and MVC group is the smallest building block you can still configure how it’s assembled. Have a look at the application’s configuration to find out how
package sample.lanterna.groovy
application {
title = 'Lanterna + Groovy'
startupGroups = ['sample']
autoShutdown = true
}
mvcGroups {
// MVC Group for "sample"
'sample' {
view = 'sample.lanterna.groovy.SampleView'
controller = 'sample.lanterna.groovy.SampleController'
}
}
1.3.2. Controller
We find that for the Java version the Controler it’s identical to the Swing
and JavaFX versions. For the Groovy one we notice that both input
and output
view components are accessed directly. We know we’ve said in the past
that a Controller should never do this but because Lanterna exposes no bind mechanism
the Groovy binding implementation would look as verbose as the Java version; we
decided to take a shortcut for demonstrations purposes.
package sample.lanterna.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonController;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonController;
import javax.annotation.Nonnull;
import javax.inject.Inject;
@ArtifactProviderFor(GriffonController.class)
public class SampleController extends AbstractGriffonController {
private SampleModel model; (1)
@Inject
private SampleService sampleService; (2)
@Inject
public SampleController(@Nonnull GriffonApplication application) {
super(application);
}
public void setModel(SampleModel model) {
this.model = model;
}
public void sayHello() { (3)
final String result = sampleService.sayHello(model.getInput());
runInsideUIAsync(new Runnable() { (4)
@Override
public void run() {
model.setOutput(result);
}
});
}
}
1 | MVC member injected by MVCGroupManager |
2 | Injected by JSR 330 |
3 | Automatically run off the UI thread |
4 | Get back inside the UI thread |
package sample.lanterna.groovy
import griffon.core.artifact.GriffonController
import griffon.metadata.ArtifactProviderFor
import javax.inject.Inject
@ArtifactProviderFor(GriffonController)
class SampleController {
FactoryBuilderSupport builder (1)
@Inject
private SampleService sampleService (2)
void sayHello() { (3)
String result = sampleService.sayHello(builder.input.text)
runInsideUIAsync { (4)
builder.output.text = result
}
}
}
1 | MVC member injected by MVCGroupManager |
2 | Injected by JSR 330 |
3 | Automatically run off the UI thread |
4 | Get back inside the UI thread |
1.3.3. Service
Again, services should not be affected by the choice of UI tookit so the following Service definitions are identical to the previous ones be saw earlier.
package sample.lanterna.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonService;
import griffon.core.i18n.MessageSource;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonService;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import static griffon.util.GriffonNameUtils.isBlank;
import static java.util.Arrays.asList;
@ArtifactProviderFor(GriffonService.class)
public class SampleService extends AbstractGriffonService {
@Inject
public SampleService(@Nonnull GriffonApplication application) {
super(application);
}
public String sayHello(String input) {
MessageSource messageSource = getApplication().getMessageSource();
if (isBlank(input)) {
return messageSource.getMessage("greeting.default");
} else {
return messageSource.getMessage("greeting.parameterized", asList(input));
}
}
}
package sample.lanterna.groovy
import griffon.core.artifact.GriffonService
import griffon.core.i18n.MessageSource
import griffon.metadata.ArtifactProviderFor
import static griffon.util.GriffonNameUtils.isBlank
@ArtifactProviderFor(GriffonService)
class SampleService {
String sayHello(String input) {
MessageSource ms = application.messageSource
isBlank(input) ? ms.getMessage('greeting.default') : ms.getMessage('greeting.parameterized', [input])
}
}
1.3.4. View
We’d expect the View to follow the same pattern we’ve seen in the previous examples,
that is, create two components that will be bound to model properties and a button
that’s connected to a Controller action. Here we see that the Java version is rather
verbose due to the fact that Lanterna has no observable UI components. This is the
reason for which we must explicitly mode the value of the input
component into
the input
Model property as soon as the button is clicked.
package sample.lanterna.java;
import com.googlecode.lanterna.gui.Window;
import com.googlecode.lanterna.gui.component.Label;
import com.googlecode.lanterna.gui.component.Panel;
import com.googlecode.lanterna.gui.component.TextBox;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonView;
import griffon.lanterna.support.LanternaAction;
import griffon.lanterna.widgets.MutableButton;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.lanterna.artifact.AbstractLanternaGriffonView;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.Collections;
@ArtifactProviderFor(GriffonView.class)
public class SampleView extends AbstractLanternaGriffonView {
private SampleController controller; (1)
private SampleModel model; (1)
@Inject
public SampleView(@Nonnull GriffonApplication application) {
super(application);
}
public void setController(SampleController controller) {
this.controller = controller;
}
public void setModel(SampleModel model) {
this.model = model;
}
@Override
public void initUI() {
Window window = (Window) getApplication()
.createApplicationContainer(Collections.<String, Object>emptyMap());
getApplication().getWindowManager().attach("mainWindow", window); (2)
Panel panel = new Panel(Panel.Orientation.VERTICAL);
panel.addComponent(new Label(getApplication().getMessageSource().getMessage("name.label")));
final TextBox input = new TextBox();
panel.addComponent(input);
LanternaAction sayHelloAction = toolkitActionFor(controller, "sayHello");
final Runnable runnable = sayHelloAction.getRunnable();
sayHelloAction.setRunnable(new Runnable() { (3)
@Override
public void run() {
model.setInput(input.getText());
runnable.run();
}
});
panel.addComponent(new MutableButton(sayHelloAction)); (4)
final Label output = new Label();
panel.addComponent(output);
model.addPropertyChangeListener("output", new PropertyChangeListener() { (3)
@Override
public void propertyChange(PropertyChangeEvent evt) {
output.setText(String.valueOf(evt.getNewValue()));
}
});
window.addComponent(panel);
}
}
1 | MVC member injected by MVCGroupManager |
2 | Create window and attach it to WindowManager |
3 | Apply component-to-model binding |
4 | Hook actions by convention |
Fortunately we can rely on the observable output
Model property to write back the
value to the output
component as soon as said property gets updated.
package sample.lanterna.groovy
import griffon.core.artifact.GriffonView
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonView)
class SampleView {
FactoryBuilderSupport builder (1)
void initUI() {
builder.with {
application(id: 'mainWindow') { (2)
verticalLayout()
label(application.messageSource.getMessage('name.label'))
textBox(id: 'input')
button(sayHelloAction) (3)
label(id: 'output')
}
}
}
}
1 | MVC member injected by MVCGroupManager |
2 | Create window and attach it to WindowManager |
3 | Hook actions by convention |
Here we can certify there’s no binding code in the View, hence why the Controller has to access UI components directly.
1.3.5. Resources
The resources file is identical to the ones found in the other applications. There’s in fact no changes brought by the choice of UI toolkit here.
name.label = Please enter your name
greeting.default = Howdy stranger!
greeting.parameterized = Hello {0}
1.4. Pivot
Apache Pivot is an open-source platform for building installable Internet applications (IIAs). It combines the enhanced productivity and usability features of a modern user interface toolkit with the robustness of the Java platform.
We decided to implement this application in the same fashion as the Lanterna application because of the same reason: Pivot UI components are not observable. You’ll notice that the Java Model is identical and there’s no Groovy Model
1.4.1. Model
package sample.pivot.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonModel;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonModel;
import javax.annotation.Nonnull;
import javax.inject.Inject;
@ArtifactProviderFor(GriffonModel.class)
public class SampleModel extends AbstractGriffonModel {
private String input; (1)
private String output; (1)
@Inject
public SampleModel(@Nonnull GriffonApplication application) {
super(application);
}
public String getInput() { (2)
return input;
}
public void setInput(String input) {
firePropertyChange("input", this.input, this.input = input); (3)
}
public String getOutput() { (2)
return output;
}
public void setOutput(String output) {
firePropertyChange("output", this.output, this.output = output); (3)
}
}
1 | Define a private field for the property |
2 | Property accessor |
3 | Property mutator must fire a PropertyChangeEvent |
1.4.2. Controller
The Pivot Controllers follow the same rules than the Lanterna ones, and as such you’ll see there are no differences between one another.
package sample.pivot.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonController;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonController;
import javax.annotation.Nonnull;
import javax.inject.Inject;
@ArtifactProviderFor(GriffonController.class)
public class SampleController extends AbstractGriffonController {
private SampleModel model; (1)
@Inject
private SampleService sampleService; (2)
@Inject
public SampleController(@Nonnull GriffonApplication application) {
super(application);
}
public void setModel(SampleModel model) {
this.model = model;
}
public void sayHello() { (3)
final String result = sampleService.sayHello(model.getInput());
runInsideUIAsync(new Runnable() { (4)
@Override
public void run() {
model.setOutput(result);
}
});
}
}
1 | MVC member injected by MVCGroupManager |
2 | Injected by JSR 330 |
3 | Automatically run off the UI thread |
4 | Get back inside the UI thread |
package sample.pivot.groovy
import griffon.core.artifact.GriffonController
import griffon.metadata.ArtifactProviderFor
import javax.inject.Inject
@ArtifactProviderFor(GriffonController)
class SampleController {
FactoryBuilderSupport builder (1)
@Inject
private SampleService sampleService (2)
void sayHello() { (3)
String result = sampleService.sayHello(builder.input.text)
runInsideUIAsync { (4)
builder.output.text = result
}
}
}
1 | MVC member injected by MVCGroupManager |
2 | Injected by JSR 330 |
3 | Automatically run off the UI thread |
4 | Get back inside the UI thread |
1.4.3. Service
Services remain constant again, what a surprise, right?
package sample.pivot.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonService;
import griffon.core.i18n.MessageSource;
import griffon.metadata.ArtifactProviderFor;
import org.codehaus.griffon.runtime.core.artifact.AbstractGriffonService;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import static griffon.util.GriffonNameUtils.isBlank;
import static java.util.Arrays.asList;
@ArtifactProviderFor(GriffonService.class)
public class SampleService extends AbstractGriffonService {
@Inject
public SampleService(@Nonnull GriffonApplication application) {
super(application);
}
public String sayHello(String input) {
MessageSource messageSource = getApplication().getMessageSource();
if (isBlank(input)) {
return messageSource.getMessage("greeting.default");
} else {
return messageSource.getMessage("greeting.parameterized", asList(input));
}
}
}
package sample.pivot.groovy
import griffon.core.artifact.GriffonService
import griffon.core.i18n.MessageSource
import griffon.metadata.ArtifactProviderFor
import static griffon.util.GriffonNameUtils.isBlank
@ArtifactProviderFor(GriffonService)
class SampleService {
String sayHello(String input) {
MessageSource ms = application.messageSource
isBlank(input) ? ms.getMessage('greeting.default') : ms.getMessage('greeting.parameterized', [input])
}
}
1.4.4. View
If you squint your eyes you’ll see that the View is almost identical to the Swing
and Lanterna Views. Besides using toolkit specific components we notice
that both input
and output
Model properties have to be explicitly bound using
the native support exposed by the toolkit.
package sample.pivot.java;
import griffon.core.GriffonApplication;
import griffon.core.artifact.GriffonView;
import griffon.metadata.ArtifactProviderFor;
import griffon.pivot.support.PivotAction;
import griffon.pivot.support.adapters.TextInputContentAdapter;
import org.apache.pivot.serialization.SerializationException;
import org.apache.pivot.wtk.*;
import org.codehaus.griffon.runtime.pivot.artifact.AbstractPivotGriffonView;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.Collections;
@ArtifactProviderFor(GriffonView.class)
public class SampleView extends AbstractPivotGriffonView {
private SampleController controller; (1)
private SampleModel model; (1)
@Inject
public SampleView(@Nonnull GriffonApplication application) {
super(application);
}
public void setController(SampleController controller) {
this.controller = controller;
}
public void setModel(SampleModel model) {
this.model = model;
}
@Override
public void initUI() {
Window window = (Window) getApplication()
.createApplicationContainer(Collections.<String, Object>emptyMap());
window.setTitle(getApplication().getConfiguration().getAsString("application.title"));
window.setMaximized(true);
getApplication().getWindowManager().attach("mainWindow", window); (2)
BoxPane vbox = new BoxPane(Orientation.VERTICAL);
try {
vbox.setStyles("{horizontalAlignment:'center', verticalAlignment:'center'}");
} catch (SerializationException e) {
// ignore
}
vbox.add(new Label(getApplication().getMessageSource().getMessage("name.label")));
TextInput input = new TextInput();
input.setName("inputField");
input.getTextInputContentListeners().add(new TextInputContentAdapter() { (3)
@Override
public void textChanged(TextInput arg0) {
model.setInput(arg0.getText());
}
});
vbox.add(input);
PivotAction sayHelloAction = toolkitActionFor(controller, "sayHello");
final Button button = new PushButton(sayHelloAction.getName());
button.setName("sayHelloButton");
button.setAction(sayHelloAction); (4)
vbox.add(button);
final TextInput output = new TextInput();
output.setName("outputField");
output.setEditable(false);
model.addPropertyChangeListener("output", new PropertyChangeListener() { (3)
@Override
public void propertyChange(PropertyChangeEvent evt) {
output.setText(String.valueOf(evt.getNewValue()));
}
});
vbox.add(output);
window.setContent(vbox);
}
}
1 | MVC member injected by MVCGroupManager |
2 | Create window and attach it to WindowManager |
3 | Apply component-to-model binding |
4 | Hook actions by convention |
package sample.pivot.groovy
import griffon.core.artifact.GriffonView
import griffon.metadata.ArtifactProviderFor
@ArtifactProviderFor(GriffonView)
class SampleView {
FactoryBuilderSupport builder (1)
void initUI() {
builder.with {
application(title: application.configuration['application.title'],
id: 'mainWindow', maximized: true) { (2)
vbox(styles: "{horizontalAlignment:'center', verticalAlignment:'center'}") {
label(application.messageSource.getMessage('name.label'))
textInput(id: 'input')
button(id: 'sayHelloButton', sayHelloAction) (3)
textInput(id: 'output', editable: false)
}
}
}
}
}
1 | MVC member injected by MVCGroupManager |
2 | Create window and attach it to WindowManager |
3 | Hook actions by convention |